Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial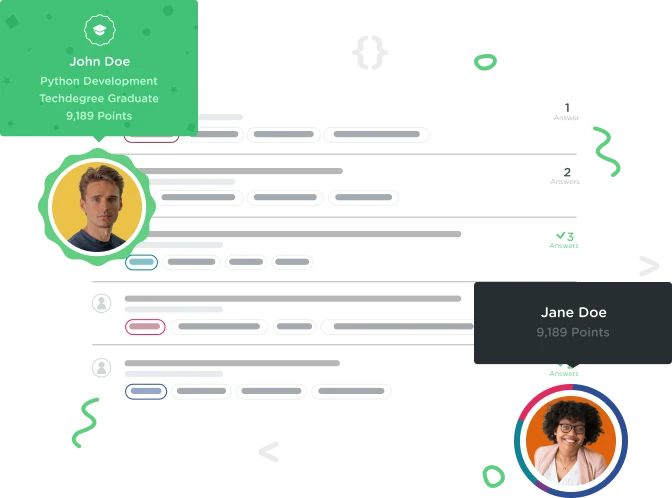
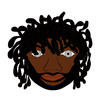
SAMUEL LAWRENCE
Courses Plus Student 8,447 PointsCode review
Hi guys, I'm at the end of the arrays lesson and Dave McFarland asked to build a quiz. We need to ask a series of question and evaluate each answer. The program should keep track of the number of questions answered correctly. After all of the questions have been answered the program should display the number of questions that were correctly answered and the number that the player got wrong.
We had to use a two-dimensional array to hold the questions and the answers, which has at least 3 questions in it. We had to use a loop to cycle through each question. Ask it and compare the user's response to the answers in the array. We had to use the prompt method to ask the question. We then had to use a conditional statement to see if the player's response matches the real answer. When the loop is done we should know how many questions were correctly answered and then print it out to the screen.
I would say I wrote a program that works at 95%. I haven't looked at the solution video yet. It took me two days to write this program and there is still one thing that isn't working for me. I can't seem to get the program to convert the user's response to all lower or upper case. Everything else works fine.
Please review my code and tell me what I'm doing wrong.
Thanks
questions = [
['What is the capital of China?', 'Beijing'],
['How many continents are there?', '7'],
['How many legs does an insect have?', '6']
];
var correct = '<h2>You got these/this question(s) correct.</h2>';
var wrong = '<h2>You got these/this question(s) wrong.</h2>';
var ask;
var total = 0;
function print(message) {
document.write(message);
}
function quiz(quest) {
correct += '<ol>';
wrong += '<ol>';
for (var i = 0; i < quest.length; i += 1) {
ask = prompt(quest[i][0]);
ask = ask.toLowerCase();
if (ask === quest[i][1]) {
correct += '<li>' + quest[i][0] + '</li>';
total += 1;
} else {
wrong += '<li>' + quest[i][0] + '</li>';
}
}
correct += '</ol>';
wrong += '</ol>';
/* This checks to see if the user got all the questions correct or wrong or if he answered some questions correctly and some wrongly*/
/* If the user got all 3 correct then the "you got these wrong" won't print to the page. If he got all 3 wrong, the "you got these correct" won't print to the page*/
if (total === quest.length) {
print('<p>You got all ' + total + ' questions correct!' + correct + '</p>');
} else if (total === 0) {
print('<p>You got ' + total + ' questions correct!' + wrong + '</p>');
} else {
print('<p>you got ' + total + ' questions right.' + '</p>');
print(correct + wrong);
}
}
quiz(questions);
Going to check out the solution video now.
1 Answer
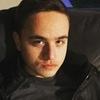
Emre Karakuz
9,162 PointsHey Samuel,
Your program is already converting user's response to lowercase. However, the correct answer that is written in questions[0][1] is 'Beijing' . Since when user enters 'Beijing', program converts it to 'beijing' and 'beijing' !== 'Beijing'. You can prevent this with changing questions[0][1] value to 'beijing'. Everything else works fine.
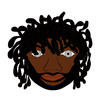
SAMUEL LAWRENCE
Courses Plus Student 8,447 PointsDUH! Idiot! I'm referring to myself Emre Karakuz . Thanks. That did it. lol
SAMUEL LAWRENCE
Courses Plus Student 8,447 PointsSAMUEL LAWRENCE
Courses Plus Student 8,447 PointsSo after looking at the solution video, I realized I did more than was asked for. That's because in the first video explaining the challenge I looked at the results on his screen and created that. The second part of the challenge asked to make the quiz more interesting by adding the other details and he suggested using adding items to an array with the pop, unshift, shift methods. So I'm going to rewrite this program using his suggested method. Please still look at this program and offer some feedback. Thanks.