Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial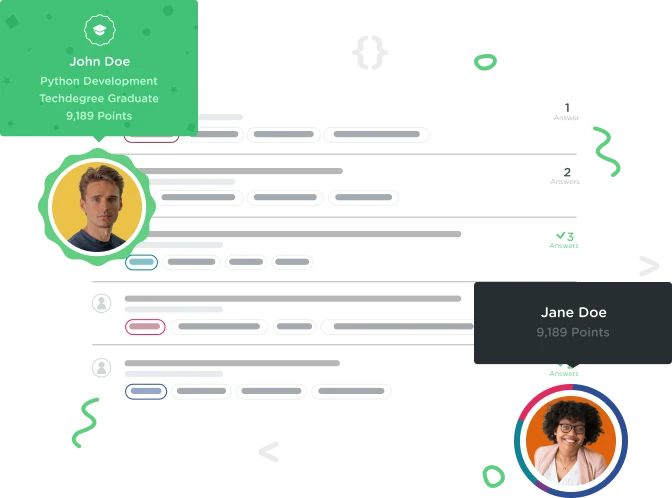

Ryan Carson
23,287 PointsCode Review: Python Dungeon Game
Would love to hear your feedback on my Dungeon Game in Python. I have a feeling I shouldn't have been using global variables all over the place and instead I should be passing in things to functions.
import random
ROOM_GRID = [(1,1), (1,2), (1,3), (1,4), (1,5),
(2,1), (2,2), (2,3), (2,4), (2,5),
(3,1), (3,2), (3,3), (3,4), (3,5),
(4,1), (4,2), (4,3), (4,4), (4,5),
(5,1), (5,2), (5,3), (5,4), (5,5)]
# Keep track of where the player has been
player_trail = []
def get_locations():
start_location = random.choice(ROOM_GRID)
monster_location = random.choice(ROOM_GRID)
door_location = random.choice(ROOM_GRID)
if monster_location == door_location or monster_location == start_location or door_location == start_location:
return get_locations()
return start_location, monster_location, door_location
def print_map():
count = 0
printed_map = []
while count < len(ROOM_GRID):
current_room = ROOM_GRID[count]
if current_room == player_location:
printed_map.append('X')
elif current_room in player_trail:
printed_map.append('.')
else:
printed_map.append('*')
if current_room[1] == 5:
printed_map.append("\n")
count += 1
print(''.join(printed_map))
# Get the player, monster and door locations
player_location, monster_location, door_location = get_locations()
# Add the player's location to their 'trail'
player_trail.append(player_location)
print("Welcome to the Dungeon!")
print("Direction keys: 'a' for left, 'd' for right, 'x' for down and 'w' for up")
print_map()
while True:
move = input("Move which direction? ")
move = move.lower()
# Move left
if move == "a" and player_location[1] > 1:
player_location = player_location[0], player_location[1]-1
# Move right
elif move == "d" and player_location[1] < 5:
player_location = player_location[0], player_location[1]+1
# Move down
elif move == "x" and player_location[0] < 5:
player_location = player_location[0]+1, player_location[1]
# Move up
elif move == "w" and player_location[0] > 1:
player_location = player_location[0]-1, player_location[1]
# Add the player's location to their 'trail'
player_trail.append(player_location)
if player_location == monster_location:
print_map()
print("Oh no! The Moster got you!")
break
if player_location == door_location:
print_map()
print("Yay! You escaped!")
break
print_map()
4 Answers
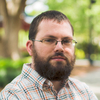
Kenneth Love
Treehouse Guest TeacherWhy are you holding onto a count
variable in print_map()
? Why not just loop through all of the members of ROOM_GRID
(which you're already doing) directly?
Also, you call print_map()
a lot. You'd probably reduce that by putting it inside your while True
loop.
I'd probably add a move_player()
function, too, that handles the moving and adding to the trail.
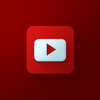
Leon Kenedy
Courses Plus Student 9,149 PointsIt looks amazing man, I will play it later. The variables will be fine.
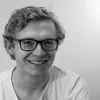
Luke Glazebrook
13,564 PointsHey Ryan!
Looks like a fairly solid program to me! Everything seems well written and I can't see why there would be any problem with your variables in a small application.
-Luke
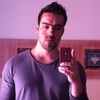
ivomiranda
30,286 PointsLol can't wait to do the last course of Python to comment on this. I'll do it as soon as I have some spare time :)
Ryan Carson
23,287 PointsRyan Carson
23,287 PointsWeird, you're right - not sure whey I have a
count
inside the print_map() function. Thanks.