Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial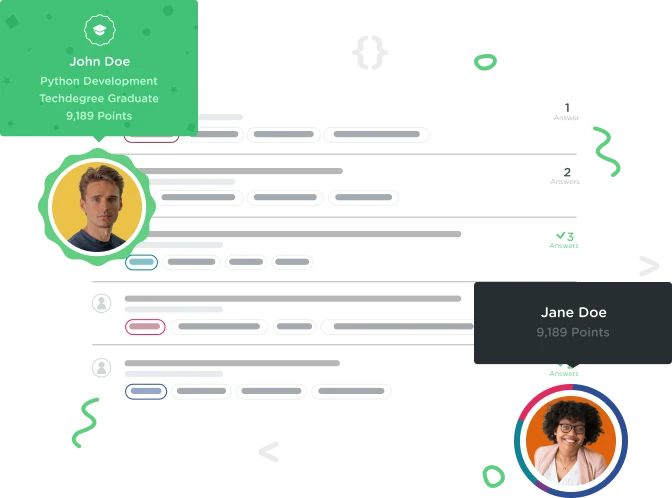

Michael Hoffmann
1,801 PointsCode runs fine in Visual Studio Code - why is it giving me an error?
class Student: name = "Michael" grade = True def praise(self): return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if self.grade > 50:
return self.praise()
if self.grade <= 50:
return self.reassurance()
Michael = Student() Michael.grade = 80 print(Michael.feedback(True))
Bummer: didnt find the praise message for grade over 50
class Student:
name = "Michael"
grade = True
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if self.grade > 50:
return self.praise()
if self.grade <= 50:
return self.reassurance()
Michael = Student()
Michael.grade = 80
print(Michael.feedback(True))
2 Answers

KRIS NIKOLAISEN
54,967 PointsSince grade
isn't an attribute you don't need self:
class Student:
name = "Michael"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if grade > 50:
return self.praise()
if grade <= 50:
return self.reassurance()
Michael = Student()
print(Michael.feedback(80))

KRIS NIKOLAISEN
54,967 Pointsgrade
isn't a boolean or attribute of the class. The numeric value for grade
passed in to the feedback method should be the value evaluated. To test try
Michael = Student()
print(Michael.feedback(80))

Michael Hoffmann
1,801 PointsThanks for your answer, Kris!
This is the code now:
class Student:
name = "Michael"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if self.grade > 50:
return self.praise()
if self.grade <= 50:
return self.reassurance()
Michael = Student()
print(Michael.feedback(80))
and this yields the following error:
File "c:/Users/micha/Documents/OOPython/student.py", line 10, in feedback
if self.grade > 50:
AttributeError: 'Student' object has no attribute 'grade'
So, what do I feed the if > return, in order to check the value 80, if I am not using an attribute?
Michael Hoffmann
1,801 PointsMichael Hoffmann
1,801 PointsThank you for being patient with me - I finally got it ;-)