Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial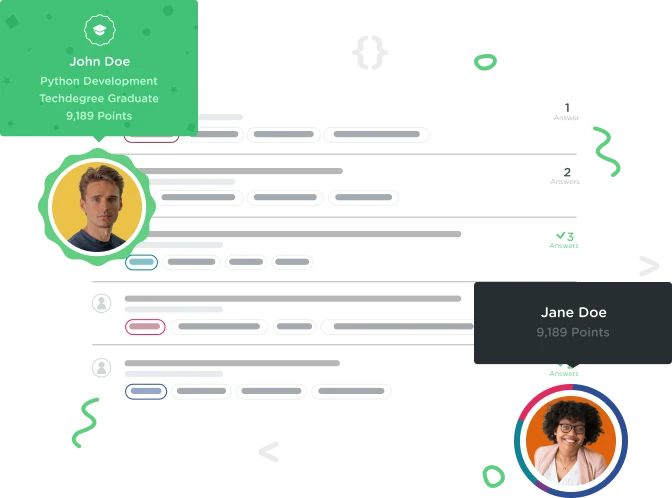

Austin Whitelaw
9,065 PointsCode seems correct, but monster_turn only executes once and then player_turn every time after that
This is my game.py code but I have been comparing it for a while to the downloaded game.py and don't see what is wrong.
import sys
from character import Character
from monster import Dragon
from monster import Goblin
from monster import Troll
class Game:
def setup(self):
self.player = Character()
self.monsters = [Goblin(), Troll(), Dragon()]
self.monster = self.get_next_monster()
def get_next_monster(self):
try:
return self.monsters.pop(0)
except IndexError:
return None
def monster_turn(self):
if self.monster.attack():
print('{} attacks you.'.format(self.monster))
if input("Dodge? Y/N: ").upper() == 'Y':
if not self.player.dodge():
self.player.hit_points -= 1
print("Dodge failed! You lost 1 hit point.")
else:
print("You dodged {}'s attack!".format(self.monster))
else:
self.player.hit_points -= 1
print("{} hit you for 1 hip point.".format(self.monster))
else:
print('{} attack failed.'.format(self.monster))
def player_turn(self):
action = input("What would you like to do? [A]ttack, [R]est, [Q]uit").upper()
if action == 'A':
print("You're attacking {}!".format(self.monster))
if self.player.attack():
if self.monster.dodge():
print("{} dodged your attack!".format(self.monster))
else:
if self.player.leveled_up():
self.monster.hit_points -= 2
else:
self.monster.hit_points -= 1
print("You hit {} with your {}!".format(self.monster, self.player.weapon))
else:
print("You missed!")
if action == 'R':
self.player.rest()
if action == 'Q':
sys.exit()
else:
self.player_turn()
def cleanup(self):
if self.monster.hit_points <= 0:
self.player.experience += self.monster.experience
print("You killed {}!".format(self.monster))
self.monster = self.get_next_monster()
def __init__(self):
self.setup()
while self.player.hit_points and (self.monster or self.monsters):
print('\n'+'='*20)
print(self.player)
self.monster_turn()
print('-'*20)
self.player_turn()
self.cleanup()
print('\n'+'+'*20)
if self.player.hit_points:
print("You win!")
elif self.monsters or self.monster:
print("You lose!")
sys.exit()
Game()
1 Answer
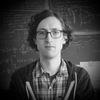
Joshua Yoerger
11,206 PointsI think you need to change the if
statements for your player actions to elif
s like so:
elif action == 'R':
self.player.rest()
elif action == 'Q':
sys.exit()
else:
self.player_turn()
Also, remember you can add syntax highlighting to your code blocks by using ```python
as your code block opening. This makes your code easier to read and debug. There is more info on posting code here
Hope that helps. Cheers.
Chris Freeman
Treehouse Moderator 68,454 PointsChris Freeman
Treehouse Moderator 68,454 PointsMoved comment to Answer. +1
Good catch. If the
action
isn't "Q", the lastelse
will causeplayer_turn
to run again, and again.