Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial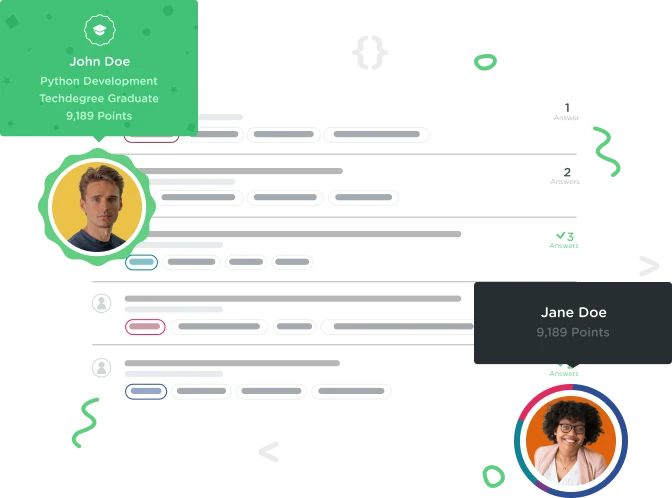

fahad lashari
7,693 PointsCode suggestion
So I completed and passed the code challenge however I feel like I didn't actually complete the challenge using the methods that are encouraged in this course. Here is my solution. Please let me know what I can do most efficiently and easily:
my_dict = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': ['Python Basics', 'Python Collections']}
def most_classes(dictionary):
if len(dictionary['Jason Seifer']) > len(dictionary['Kenneth Love']):
return 'Jason Seifer'
else:
return 'Kenneth Love'
def num_teachers(dictionary):
return len(dictionary)
def stats(dictionary):
list = []
for teacher in dictionary:
list.append([teacher, len(dictionary[teacher])])
return list
def courses(dictionary):
list = []
for course_list in dictionary.values():
for course in course_list:
list.append(course)
return list
most_classes(my_dict)
num_teachers(my_dict)
stats(my_dict)
courses(my_dict)
Please mind the wording in the code. My life is pretty dull so I do what I can to have chuckle from time to time :)
2 Answers
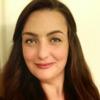
Jennifer Nordell
Treehouse TeacherHi there fahad lashari! Congratulations on completing the challenge!
I would say that your code looks very "modular" meaning that you are keeping the functions very specific to one particular task. This is actually a great thing. But there is one function here that I feel like could use improvement:
def most_classes(dictionary):
if len(dictionary['Jason Seifer']) > len(dictionary['Kenneth Love']):
return 'Jason Seifer'
else:
return 'Kenneth Love'
This will give you your desired result. However, it only applies to this data set. What if there had been 5 teachers or 100? And what if none of them were named Jason Seifer or Kenneth Love? Ideally, what you'd like to see here is a function that can take any number of teachers, go through them all, and return the teacher that has the most classes. You'll likely need a variable to hold the current max number of classes and the teacher's name.
For example, let's start a max_classes variable equal to 0. And teacher equal to an empty string. Then look at the first teacher. Let's say it's Dave McFarland with 4 classes. We would record into the max_classes and keep the name Dave McFarland. We move down our list and we don't find another teacher with more than 4 classes until we get to Nick Petit who has 10. At this point, we set our max_classes to 10 and the name to Nick Petit and continue to see if there's someone who has even more than that. This code will allow us to return the teacher of any given data set who has the most classes instead of just these two given in my_dict.
Hope this helps!

fahad lashari
7,693 PointsOops sorry I sincerely apologize. Won't happen again
Michael Hulet
47,913 PointsMichael Hulet
47,913 PointsJust letting you know, I edited this post to weed out a lot of profanity, and also to prettify the markdown a little bit. Please don't use any profanity in your questions or your code in the Treehouse Community