Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial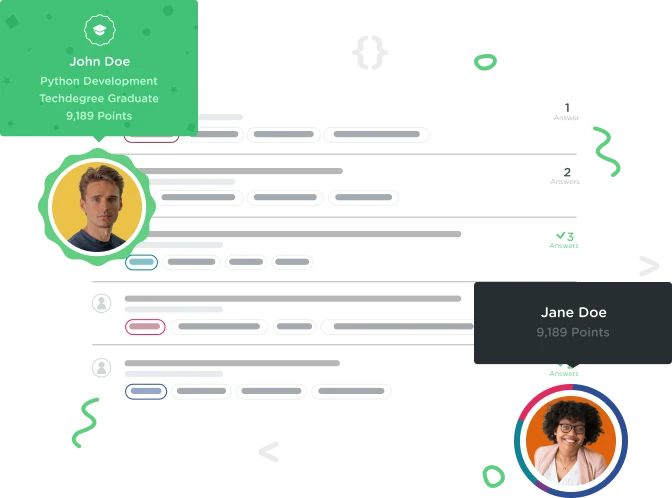

Kenneth Dubroff
10,612 PointsCode won't compile, no errors show in preview
Maybe I'm not understanding the challenge correctly since I can't think of an instance where assigning the value of result instead of a bool value would be useful, but I've tried it that way as well, and get the same compiler error. I tested my code in a playground and it works as I would expect
// Enter your code below
let value = 200
let divisor = 5
let someOperation = 20 + 400 % 10 / 2 - 15
let anotherOperation = 52 * 27 % 200 / 2 + 5
// Task 1 - Enter your code below
let result = value % divisor
// Task 2 - Enter your code below
if result == 0 {
let isPerfectMultiple = result
}
5 Answers

Kenneth Dubroff
10,612 PointsFor the record, doing that way doesn't compile either. Works fine in Xcode, but not in the treehouse compiler. Again, no errors in the preview Edit: This was in response to the if/else syntax... the compiler didn't like it that way even though Xcode is fine with it
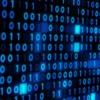
Alexander Davison
65,469 PointsThe variable isPerfectMultiple
is supposed to contain a boolean.
It seems that you are checking a condition, and if the condition is true, assigning the variable to result
, which is an integer.
If you read the challenge carefully, you'll see that mistake.
Here's a hint:
if (result == 0) {
// Assign isPerfectMultiple to true
} else {
// Assign isPerfectMultiple to false
}
Tip: Since
result == 0
returns a boolean itself, you can also set isPerfectMultiple
to that condition instead of doing a if/else
condition.

Kenneth Dubroff
10,612 PointsThank you for the help, however, I've read the challenge carefully several times, and do not see my mistake. I understand what your code will output, and how to write a comparative statement. I understand that doing it that way makes sense, and assigning the result to isPerfectMultiple makes zero sense in any application I can think of. BUT... the challenge explicitly states:
"Compare the value of result to 0 using the equality operator and assign the resulting value to a constant named isPerfectMultiple."
which is what I've done. I'm not seeing how I'll be able to continue the course if ambiguity is the name of the game in challenges
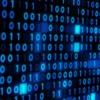
Alexander Davison
65,469 PointsYes, you have misunderstood this statement:
Compare the value of result to 0 using the equality operator and assign the resulting value to a constant named isPerfectMultiple.
It may be confusing because it seems like "the resulting value" is the value of
result
.
The "resulting value" is actually the boolean that "result == 0" returns.

Kenneth Dubroff
10,612 Pointsthe result of the constant result is an Integer - 0
0 may also be a boolean of false, but only if we make it one by assigning a boolean to isPerfectMultiple. The challenge is not asking to assign a boolean value to isPerfectMultiple. It is explicitly asking for the result to be contained in isPerfectMultiple.
I immediately arrived at the conclusion I would do it the way you're saying to do it in a real life scenario, but that is not what the challenge asks for
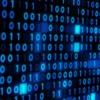
Alexander Davison
65,469 PointsYou're actually wrong, since this passes:
let isPerfectMultiple = result == 0

Kenneth Dubroff
10,612 PointsYes, that works thanks. I've never seen that syntax before.. I didn't realize you could store a comparative check in a constant/variable like that.
That's much less clunky than if/else syntax!
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsYou should add that code below the code you wrote. This is the code that should be appearing in the editor:
Kenneth Dubroff
10,612 PointsKenneth Dubroff
10,612 PointsI'm trying to mark this as best answer, but I only have the option on my own comments lol