Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial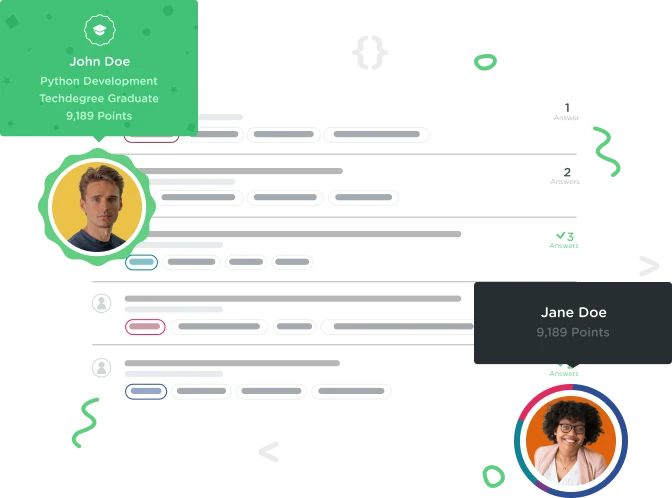

Ali Bouland
3,479 PointsCode won't work..
Any idea why? Probably something very simple..
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
class Car: Vehicle {
var numberOfSeats = 4
override init(withDoors : Int, andWheels: Int){
super.init(withDoors: withDoors, andWheels: andWheels)
}
}
var someCar = Car(withDoors:2, andWheels: 4)
// Enter your code below
2 Answers
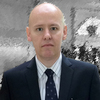
Nathan Tallack
22,160 PointsAli, you are overthinking. When you are subclsasing, you can avoid declaring an initializer if your properties in your subclass are optional or have default values. In this case your Car.numberOfSeats has a default value. So if you do not delcare an initializer you can make use of the parent class initializer without any extra effort. :)
Of course this means you can't initialize a different numberOfSeats when you are making your Car, but you can go ahead and change that poperty later after you have your object created. :)
So your code would look like this.
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
class Car: Vehicle {
var numberOfSeats = 4
}
let someCar = Car(withDoors:2, andWheels: 4)

Reed Carson
8,306 Pointsfor your override init you have deviated from the challenge parameters. you need
override init(withDoors doors: Int, andWheels wheels: Int) {
super.init(withDoors: doors, andWheels: wheels)
}
you have to be very careful about being specific for the code challenges, which is true to coding in the real world as well
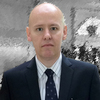
Nathan Tallack
22,160 PointsThey are trying to teach you good coding practice too. By using the external names that are more descriptive it helps the developer that is using your class in the future by offering "natural language" for the parameters. You'll notice that being used alot by Apple's own frameworks.
Of course, you wont want to be using the big long parameter names within your method, so the internal name will be nice and short (but still descriptive) allowing you to code a little cleaner.
Ali Bouland
3,479 PointsAli Bouland
3,479 PointsWhat I don't understand is this part:
init(withDoors doors: Int, andWheels wheels: Int)
why don't we just write: init(doors: Int, wheels: Int). What's the benefit of "withDoors doors" compared to just "doors"?