Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial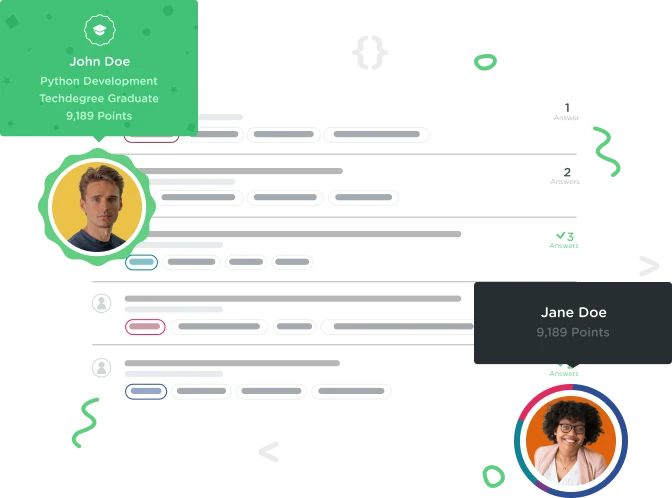

Jacob Richardson
2,969 PointsCode works, but challenge fails
With or without the bottom section of code for the top item of list, the code I have still fails the challenge.
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let li = event.target.parentNode;
let nextLi = li.previousElementSibling;
let ul = li.parentNode;
let lastLi = ul.lastElementChild;
if(nextLi){
let p = nextLi.firstElementChild;
p.className = "highlight";
}
if(!nextLi){
let p = lastLi.firstElementChild;
p.className = "highlight";
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
3 Answers

Clayton Perszyk
Treehouse Moderator 48,850 Pointsyou are doing too much; all you need to do is:
- check if element clicked is a button.
- get the previous sibling element from the target passed in.
- add a class of 'highlight'.
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') { // 1. check if button
let p = // 2. get previous sibling and assign it to variable
p.className = "highlight"; // 3. set p.className to 'highlight'
}
}
});
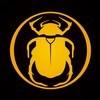
rydavim
18,814 PointsI think your answer is more complicated than what they're looking for.
Additionally, it looks like your solution is highlighting the paragraph before the button before it. They want you to highlight the paragraph immediately preceding the button that is clicked. For example, clicking on the third button should highlight the text that says Event Listening.
let li = event.target.parentNode; // No need to grab the parent node...
let nextLi = li.previousElementSibling; // ...or the list items.
let ul = li.parentNode;
let lastLi = ul.lastElementChild; // So you won't need to use children.
// If you use previousElementSibling you won't need to do if checks.
if(nextLi){
let p = nextLi.firstElementChild;
p.className = "highlight";
}
if(!nextLi){
let p = lastLi.firstElementChild;
p.className = "highlight";
}
Take a look at the second video in the series - Using previousElementSibling and insertBefore - for a refresher on this selector. The MDN page is also helpful.
// e.target is a shortcut for the event target (see listener)
// previousElementSibling grabs the sibling immediately before the target.
// You've already got className in your solution, use that here!
e.target.previousElementSibling.className = "highlight";
Hopefully that helps, but please let me know if you have any questions or if there's anything I can clarify. Happy coding!

KRIS NIKOLAISEN
54,971 PointsDid you use the preview option to check your code? When you click a button the immediately preceding paragraph should highlight. So for example if I click on the first (top) button then 'Element Selection' should highlight. With your code however the last paragraph 'Dom Traversal' highlights.