Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial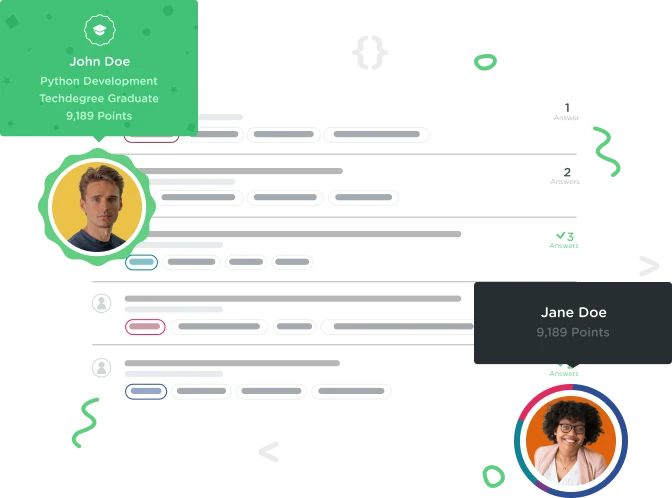

David Pollard
4,324 PointsCode works in Python, but doesn't seem to satisfy the answer required ?
Create a function named combiner that takes a single argument, which will be a list made up of strings and numbers.
Return a single string that is a combination of all of the strings in the list and then the sum of all of the numbers. For example, with the input ["apple", 5.2, "dog", 8], combiner would return "appledog13.2". Be sure to use isinstance to solve this as I might try to trick you.
def combiner(*listofthings):
num = 0.0
string = ""
for thing in listofthings:
if isinstance(thing, str):
# concat string
string = string + thing
elif isinstance(thing, (int,float)):
# add numbers
num = num + thing
return (string, num)
3 Answers
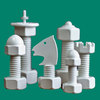
Steven Parker
231,269 PointsThe argument given will already be a list, so you don't need to "splat" operator (*).
Then, this code is returning a tuple containing a string and a number., but the challenge expects a single string. Convert the number to a string and concatenate it with the other string.
I'll bet you can do it now without a code spoiler.

jhon white
20,006 Pointsdef combiner(listofthings):
num = 0.0
string = ""
for thing in listofthings:
if isinstance(thing, str):
# concat string
string = string + thing
elif isinstance(thing, (int,float)):
# add numbers
num = num + thing
return string + str(num)
the input should be a list, and the return should be combin the strings and numbers.
Good luck :)

David Pollard
4,324 PointsThanks chaps. "Return a single string" - should have read the question better !!! :-)
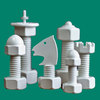
Steven Parker
231,269 PointsDavid Pollard — Glad to help. You can mark the question solved by choosing a "best answer".
And happy coding!