Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial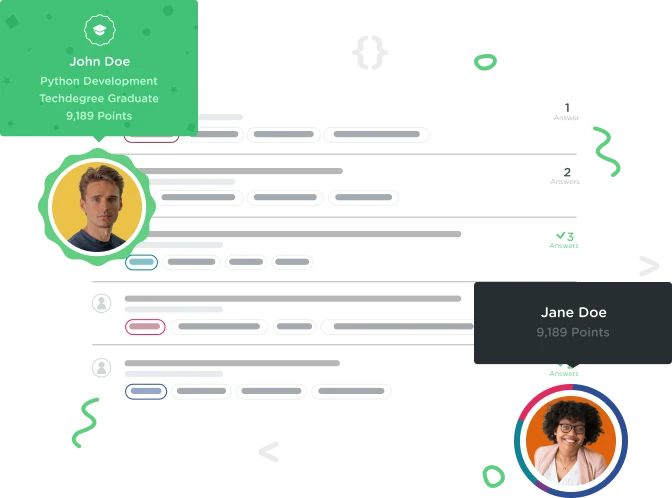
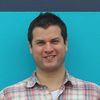
Sergio Alen
26,726 PointsCode works in xcode playground but not in the challenge
Not sure what I'm doing wrong, I keep getting the error "Make sure your second argument has the external name transformation and type (T) -> U", it works fine in Xcode but it doesnt get accepted in the challenge.
Here's my code:
func map<T, U>(array: [T], transformation: ([T]) -> [U] ) -> [U] {
return transformation(array)
}
func toSquare(_ arrayToSquare: [Int]) -> [Int] {
var returnArray = [Int]()
for i in arrayToSquare {
returnArray.append(i * i)
}
return returnArray
}
var challengeArray = [1,2,3,4,5]
map(array: challengeArray, transformation: toSquare)
2 Answers
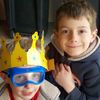
Chris Stromberg
Courses Plus Student 13,389 PointsYou need to check your code here:
func map<T, U>(array: [T], transformation: ([T]) -> [U] ) -> [U] {
return transformation(array)
}
The transformation argument type does not match what the challenge asked for.
transformation: ([T]) -> [U]
Is not the same type as :
transformation: (T) -> U
Also the challenge is asking you to create a func with two generic parameters:
In the editor define a function named map with two generic type parameters, T and U. The function takes two arguments - array of type [T], and transformation of type (T) -> U. The function returns an array of type U.
The goal of the function is to apply the transformation operation on the array passed in as argument to return a new array containing the values transformed.
Within your func you need to loop thru the generic array [T] and apply a transformation to each element. The type (T) -> U would be applied to each of your elements.
func map<T, U>(array: [T], transformation: (T) -> U) -> [U] {
var transformedArray = [U]()
for i in array {
// Here we take an array and append the result of (T) -> U for each element
// in the array provided.
transformedArray.append(transformation(i))
}
// Then we return the new array of generic [U]
return transformedArray
}

Deysha Rivera
5,088 PointsThank you for your explanation. I'm not sure how I was supposed to know from the description that I needed to use a for in loop to answer this. I don't think the clues were super clear on how to write the body of the function.
Sergio Alen
26,726 PointsSergio Alen
26,726 PointsLegend. Thanks Chris