Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial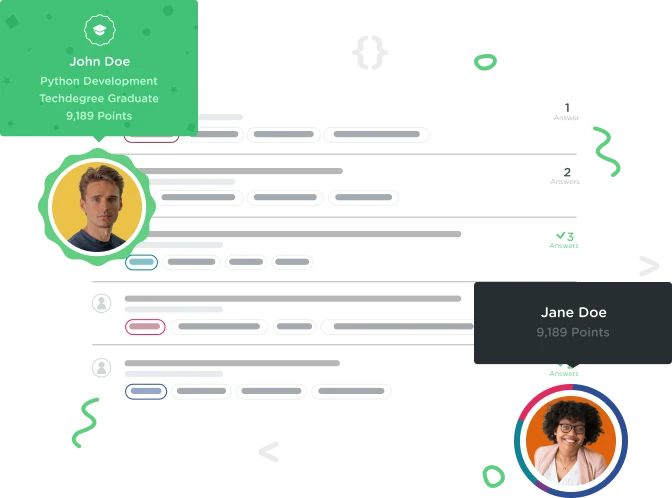

Uriahs Victor
676 PointsCode works two ways?
I'm not sure why but both of the following code seems to work in the Team Treehouse workspace, pay attention to the private tags array:
In recipes.php:
<?php
class Recipe{
private $title;
private $ingredients = array();
private $instructions = array();
private $yield;
private $tags = array();
private $source = "Alena Holligan";
private $measurements = array(
"tsp",
"tbsp",
"cup",
"oz",
"lb",
"fl oz",
"pint",
"quart",
"gallon",
);
public function setTitle($title){
$this->title = ucwords($title);
}
public function getTitle(){
return $this->title;
}
public function addIngredient($item, $amount = null, $measure = null){
if ($amount != null && !is_float($amount) && !is_int($amount)){
exit("The amount must be a float: " . gettype($amount) . " $amount given");
}
if ($measure != null && !in_array(strtolower($measure), $this->measurements)){
exit("Please enter a valid measurement: " . implode(", ", $this->measurements));
}
$this->ingredients[] = array(
"item" => ucwords($item),
"amount" => $amount,
"measure" => strtolower($measure)
);
}
public function getIngredients(){
return $this->ingredients;
}
public function addTag($tag){
$this->tags[] = strtolower($tag);
}
public function getTags(){
return $this->tags;
}
public function addInstruction($string){
$this->instructions[] = $string;
}
public function getInstructions(){
return $this->instructions;
}
public function displayRecipe(){
return $this->title . " by " . $this->source;
}
}
Cookbook.php
<?php
include "classes/recipes.php";
$recipe1 = new Recipe();
//$recipe1->source = "Grandma Holligan";
$recipe1->setTitle("my first recipe");
$recipe1 ->addIngredient("egg", 1);
$recipe1 ->addIngredient("flour", 2, "cup");
$recipe2 = new Recipe();
//$recipe2->source = "Betty Crocker";
$recipe2->setTitle("my second recipe");
echo $recipe1->getTitle();
foreach($recipe1->getIngredients() as $ing){
echo "\n" . $ing["amount"] . " " . $ing["measure"] . " " . $ing["item"];
};
$recipe1->addInstruction("This is my first instruction");
$recipe1->addInstruction("This is my second instruction");
echo implode("\n", $recipe1->getInstructions());
$recipe1->addTag("Breakfast");
$recipe1->addTag("Main Course");
echo implode(", ", $recipe1->getTags());
//echo $recipe1->displayRecipe();
//echo $recipe2->displayRecipe();
If I run this it executes fine and adds the tags, but if i change the Private tag property:
private $tags = array();
to
private $tag = array();
It seems to still work just fine...how can it still work fine if in the getter and setter we're still referencing
$this->tags[]