Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial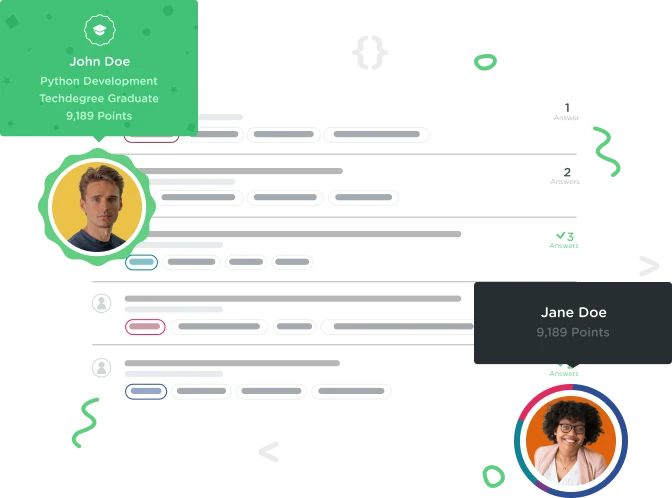

Alex Crump-Haill
7,819 PointsCode works when run on console, but doesn't pass challenge. Any ideas why?
As above, the code works when run in console workspaces but does not pass the code challenge. Why do you think this is? Do you think it is because the challenge is not asking for the use of a count and while loop? Thanks.
import random
integer = random.randint(1, 50)
iterable = list(range(50))
random_list = []
def nchoices(integer, iterable):
count = integer
while count > 0:
random_value = random.choice(iterable)
random_list.append(random_value)
count -= 1
print(random_list)
nchoices(integer, iterable)
3 Answers
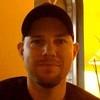
Jeremy Hill
29,567 PointsHere is what I did:
import random
def nchoices(iterable, num):
items = []
count = 0
while count < num:
items.append(iterable[random.randint(0, len(iterable))])
count += 1
return items
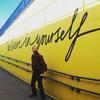
Matthew Hill
7,799 PointsYou've declared your list outside of your function, and then you're printing it, this isn't really what the question is asking you to do. You need to return a list of randomly selected items. Therfore the list needs to be created/appended within your function and then sent back. Matt

Alex Crump-Haill
7,819 Pointsimport random
integer = random.randint(1, 50) iterable = list(range(50))
def nchoices(integer, iterable): count = 0 random_list = [] while count < integer: random_value = random.choice(iterable) random_list.append(random_value) count += 1 return(random_list)
^ I have fixed that and created/appended within function but how come it still doesn't pass? Thanks : )
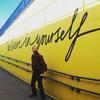
Matthew Hill
7,799 PointsHi Alex, hard to see what's going on without your response properly formatted but try removing your declarations for integer and iterable, these will be given to you, you don't need brackets around your return value, and double check your formatting. If this still doesn't work can you post your error code? Cheers, Matt
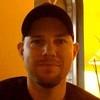
Jeremy Hill
29,567 PointsIf it works in workspace but not the challenge then it is probably because you didn't follow the instructions exactly as they have it.