Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial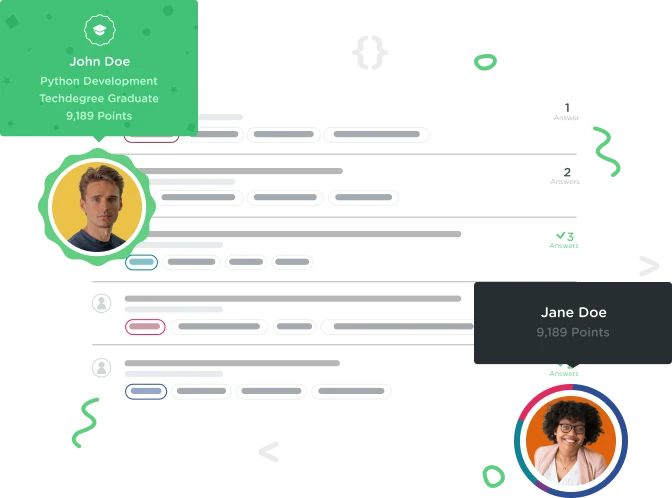

Yuval Blass
18,134 PointsCodeFights challenge
Hi everyone, I tried to solve this (https://codefights.com/interview-practice/task/pMvymcahZ8dY4g75q/) challenge, and I have no idea why my code isn't working. I tried to debug it with console.log function, and I have seen something weird; Why the first console.log(nArray) isn't the same as to the seconde one?
function firstDuplicate(a) {
const nArray = a;
console.log(nArray);
var sorted_arr = a.sort();
console.log(nArray);
var results = [];
for (var i = 0; i < a.length - 1; i++) {
if (sorted_arr[i + 1] == sorted_arr[i]) {
results.push(sorted_arr[i]);
}
}
if (results.length == 1) return results[0];
if (results.length > 1) {
var index = nArray.lastIndexOf(results[0]);
var num = results[0];
for (var j = 1; j < results.length; j++) {
if (nArray.lastIndexOf(results[j]) < index) {
num = results[j];
index = nArray.lastIndexOf(results[j]);
}
}
return num;
}
return -1;
}
firstDuplicate([2, 3, 3, 1, 5, 2]);
Thanks in advanced, Yuval Blass.
2 Answers
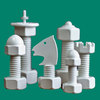
Steven Parker
231,007 PointsThe log outputs differ because the array gets sorted between them.
In an assignment of an array, the array is not copied but the new variable becomes a reference to the same array. So when "a.sort()
" is performed between the two log functions, the array that nArray refers to is changed.
If you want to copy an array instead of creating a new reference to it, a common technique is to use the slice function with no argument:
const nArray = a.slice();

Yuval Blass
18,134 PointsHi Steven,
How can I create a variable without a reference to the array? How can I make the first four lines works as I want them to be?
Here is the challenge description:
Note: Write a solution with O(n) time complexity and O(1) additional space complexity, since this is what you would be asked to do during a real interview.
Given an array a that contains only numbers in the range from 1 to a.length, find the first duplicate number for which the second occurrence has the minimal index. In other words, if there are more than 1 duplicated numbers, return the number for which the second occurrence has a smaller index than the second occurrence of the other number does. If there are no such elements, return -1.
Example
For a = [2, 3, 3, 1, 5, 2], the output should be firstDuplicate(a) = 3.
There are 2 duplicates: numbers 2 and 3. The second occurrence of 3 has a smaller index than than second occurrence of 2 does, so the answer is 3.
For a = [2, 4, 3, 5, 1], the output should be firstDuplicate(a) = -1.
Thanks, Yuval Blass.
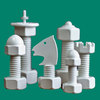
Steven Parker
231,007 PointsI updated my answer to cover this question also.
In future questions, when posting about a challenge it's helpful to provide a link to the challenge page.
Steven Parker
231,007 PointsSteven Parker
231,007 PointsSome authorization issue prevents your link from being viewed.