Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial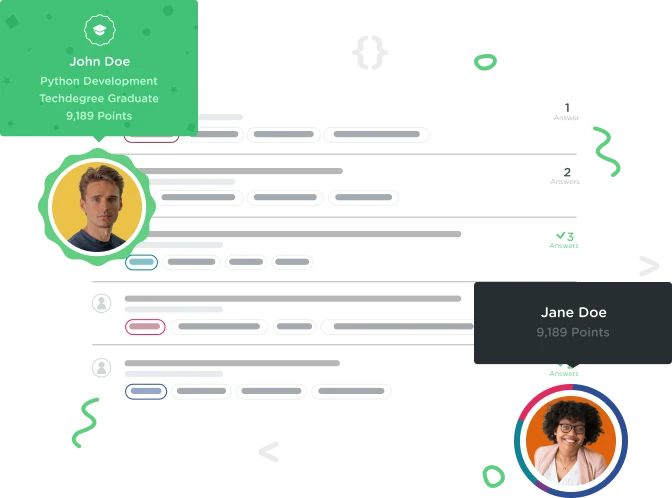

Drew Rollins
694 PointsCoding challenge: try and except.
Any insight as to why this doesn't work would be greatly appreciated!
def add (win, lose):
try:
count = int(input("Enter a number: "))
except ValueError:
return None
else:
return (float(win)+float(lose))
Thank you!
1 Answer
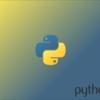
Kevin Faust
15,353 PointsWe dont take in a user input. The numbers will be passed in for us automatically
def add (win, lose):
try:
win = float(win)
lose = float(lose)
except ValueError:
return None
else:
return win+lose
So in your try block, you want to see if you can convert the passed in numbers to floats. If you can, then the rest of the try block will run and the float values will be reassigned to the variable, then we return it. Otherwise we return None
What you did also works. This would work as well:
def add (win, lose):
try:
float(win)
float(lose)
except ValueError:
return None
else:
return (float(win)+float(lose))
We dont reassign the variables passed in but rather we just check if any error will be thrown in our try block and then we do the converting in our else statement
Drew Rollins
694 PointsDrew Rollins
694 PointsThank you! Very well explained!