Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial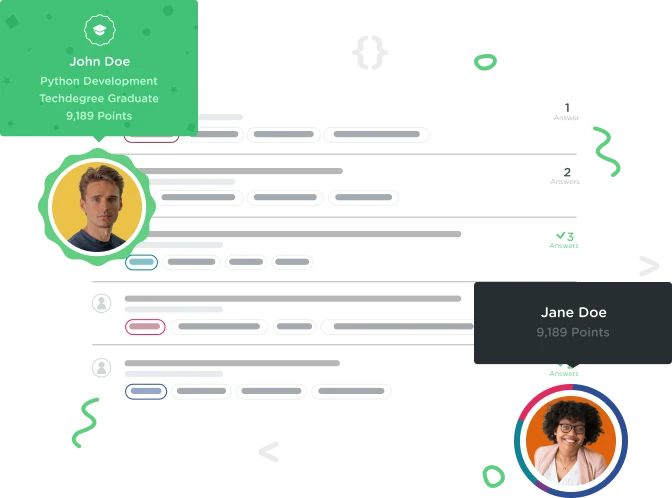

Lorrian Landicho
278 PointsCoercing integer
As part of the FizzBuzz name = input("Please enter your name: ") number = input("Please enter a number: ")
TODO: Make sure the number is an integer
number = int(number)
treehouse:~/workspace$ python challenge.py
Please enter your name: Sam
Please enter a number: 4.5
Traceback (most recent call last):
File "challenge.py", line 5, in <module>
number = int(number)
ValueError: invalid literal for int() with base 10: '4.5'
Why did the coercion to integer not work?
2 Answers

Kent Åsvang
18,823 PointsThe built-in function input() returns the input as a string. Trying to convert the string '4.5' to an integer won't work, but you can turn it into a float():
number = input("Enter a number ") # Take a number from the user
number = float(number) # Convert it to a float
A float can in turn be converted into an int, but remember that this will trim away all the decimals. int(4.5) -> 4
number = '4.5' # prints '4.5'
float(number) # prints 4.5
int(float(number)) # prints 4
Hope this helped.

Lorrian Landicho
278 PointsThe entered value 4.5 was a string, correct? And that is the reason I was coercing it to an integer.
number = "4.5" number=int(float(number))
Why is the string 4.5 can be coerced to a float, and not to integer?

Kent Åsvang
18,823 PointsIf you call int() on a string decimal like '4.5', you are implicitly calling a float() first, which is not the pythonic way. Python is aiming to be a literal and readable language, therefore int() can only coerce a string('4') or a float('45.5').
Be aware of the fact that the int() function doesn't round the float you are passing to it, it just truncates it - removes everything after the decimal point.

Carlos Vargas
1,316 PointsThe solution I came up with to this problem was to coerce the input string into a float, round the float, and coerce the float to an integer. This allowed for accurate rounding to the nearest integer.
number = int(round(float(number)))
Mike D
2,792 PointsMike D
2,792 Points4.5 is a float not an integer. So, it throws a ValueError exception because it's expecting an integer, but receiving a float instead.
If the goal is to only accept integers, consider using a try/except block, so it forces the user input to only accept int's and not break when undesired input is entered.