Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial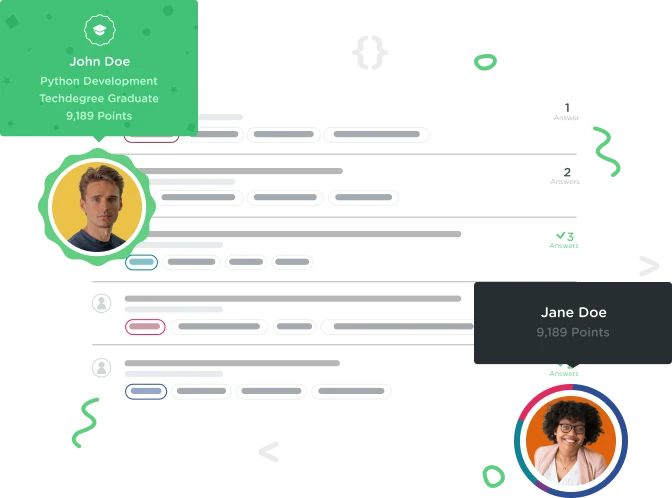
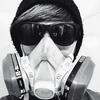
Gavin Hobbs
5,205 PointsCoin, Coins, coin, and coins--What's the difference?
I'm having a hard time figuring out what for coin in coins
is doing and referring to. Could someone possibly explain this?
enum Coin: Double {
case penny = 0.01
case nickel = 0.05
case dime = 0.10
case quarter = 0.25
}
let coins: [Coin] = [.penny, .nickel, .dime, .dime, .quarter, .quarter, .quarter]
func sum (having coins: [Coin]) -> Double {
var total: Double = 0
for coin in coins {
total += coin.rawValue
}
return total
}
sum(having: coins)
Thanks!
- Gavin
2 Answers

Greg Kaleka
39,021 PointsHi Gavin,
There's a lot going on here. I've renamed things - let me know if this helps:
enum CoinType: Double {
case penny = 0.01
case nickel = 0.05
case dime = 0.10
case quarter = 0.25
}
let myOwnCoinArray: [CoinType] = [.penny, .nickel, .dime, .dime, .quarter, .quarter, .quarter]
func sum (having passedInValueThatCanBeAnyCoinArray: [CoinType]) -> Double {
var total: Double = 0
for specificCoin in passedInValueThatCanBeAnyCoinArray {
total += specificCoin.rawValue
}
return total
}
sum(having: myOwnCoinArray)
Note: this is ridiculous, and you would never name things like this... but hopefully it helps illustrate what's what.
Cheers
-Greg
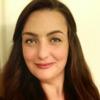
Jennifer Nordell
Treehouse TeacherHi there! I'm guessing we can agree that coins
is a constant that contains an array of enum values. This for loop is saying for every item in this array, we're going to assign it to a temporary variable named coin
. The first iteration of the loop, coin
will be equal to .penny
and we get the raw value of that which is 0.01 and add it to sum. This will keep a running count. Our second iteration, coin
will be equal to .nickel
as it moves on to the next item and we add the raw value of that, 0.05. At this point, sum is equal to 0.06. This will continue until we run out of items in the coins
array. You could have named that variable coin
just about anything, but we named it something that makes sense. It might be helpful for you to think of it instead as individualCoin
. Then we could say for individualCoin in coins
.
Hope that clarifies things!