Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial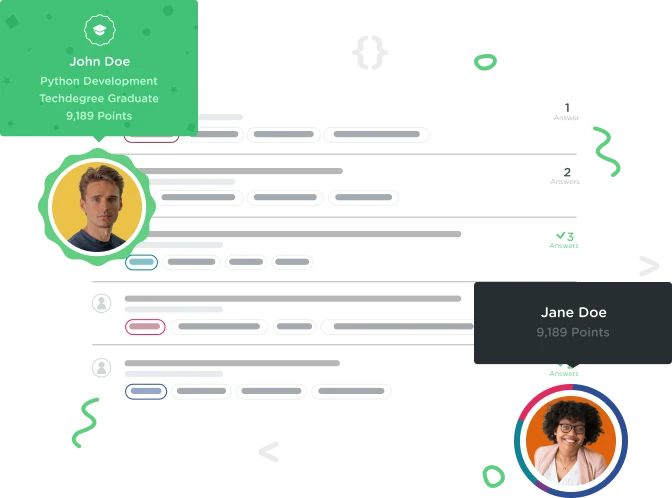
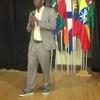
Setfree Shonhai
4,292 PointsCollection objects
Create a public method named filterHouseColor that accepts a single parameter named $color.
After going through with the code below, now l have found myself stuck, please help.
<?php class Subdivision { public $houses = []; // Same thing as array() public function filterHouseColor($color) { $lots = []; // just a temporary array
// Looks like this is where you got lost
// In order to access the $houses array, you need to do $this->houses because $houses is a property on Subdivision
// This foreach loop loops through the $houses array
// So each cycle, $house contains a $house object found in $houses
foreach ($this->houses as $house) {
// Remember: you can only access $roof_color and $wall_color from the $house object
if ($house->roof_color === $color || $house->wall_color === $color) {
$lots[] = $house->lot; // Here, we append the value of each $house's $lot property to that temporary array up there named $lots
}
}
return $lots; // Finally, we return $lots
} } ?>
<?php
// add code below this comment
class Subdivision {
public $houses = []; // Same thing as array()
public function filterHouseColor($color) {
$lots = []; // just a temporary array
// Looks like this is where you got lost
// In order to access the $houses array, you need to do $this->houses because $houses is a property on Subdivision
// This foreach loop loops through the $houses array
// So each cycle, $house contains a $house object found in $houses
foreach ($this->houses as $house) {
// Remember: you can only access $roof_color and $wall_color from the $house object
if ($house->roof_color === $color || $house->wall_color === $color) {
$lots[] = $house->lot; // Here, we append the value of each $house's $lot property to that temporary array up there named $lots
}
}
return $lots; // Finally, we return $lots
}
}
?>
2 Answers
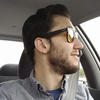
Benjamin Larson
34,055 PointsOh man, so close! Your problem is at this line:
<?php
$lots[] = $house->lot;
You want to assign the entire $house object, not a specific property of it.
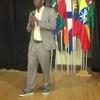
Setfree Shonhai
4,292 PointsThanks so much l now get it, it worked.
Setfree Shonhai
4,292 PointsSetfree Shonhai
4,292 PointsBenjamin thanks a lot, but my code passed on the first stage, now l am stuck on the second stage. find the question to the challenge below-
Create a public method named filterHouseColor that accepts a single parameter named $color.
Benjamin Larson
34,055 PointsBenjamin Larson
34,055 PointsSetfree Shonhai I was referring to the second stage as well. The line I posted is contained in that filterHouseColor method. You have everything written correctly except when your conditional matches a house object with the appropriate color, that entire house object needs to be assigned to the $lots[] array. The way you had it, it was only assigning the "lot" property of a given house object (which does not even exist, in this example).