Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial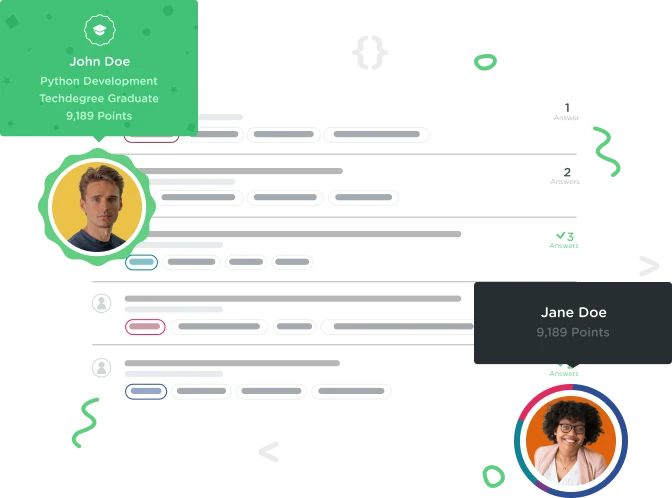

Jack Chaffin
Courses Plus Student 4,462 PointsCollections.sort method not being used in lambdas?
So... I don't quite understand why we're putting the whole collections.sort method in either of the lambda examples. Java doesn't seem to be using them. Here's my code:
package com.teamtreehouse.lambdas;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
public class Main {
public static void usingAnonymousInlineClass() {
List<Book> books = Books.all();
// Collections.sort(books, new Comparator<Book>() {
// @Override
// public int compare(Book b1, Book b2) {
// return b1.getTitle().compareTo(b2.getTitle());
// }
// });
for (Book book : books) {
System.out.println(book);
}
}
public static void usingLambdasInLongForm() {
List<Book> books = Books.all();
Collections.sort(books, (Book b1, Book b2) -> {
return b1.getTitle().compareTo(b2.getTitle());
});
books.forEach(book -> System.out.println(book));
}
public static void usingLambdasInShortForm() {
List<Book> books = Books.all();
//Collections.sort(books, (b1, b2) -> b1.getTitle().compareTo(b2.getTitle()));
books.forEach(book -> System.out.println(book));
}
public static void main(String[] args) {
usingLambdasInShortForm();
}
}
Notice how I commented out the Collections.sort lambda method in usingLamdasInShortForm()? I did that because the IDE was giving me an indication that the code wasn't being executed. Commenting it out here's the results in the terminal:
"C:\Program Files\Amazon Corretto\jdk11.0.10_9\bin\java.exe" "-javaagent:C:\Program >Files\JetBrains\IntelliJ IDEA Community Edition >2020.3.2\lib\idea_rt.jar=54466:C:\Program Files\JetBrains\IntelliJ IDEA Community >Edition 2020.3.2\bin" -Dfile.encoding=UTF-8 -classpath >C:\Users\jchaf\Downloads\lambdas\lambdas-start\Lambdas\out\production\Lambdas com.teamtreehouse.lambdas.Main
Book{mTitle='Functional Programming in Java', mAuthor='Venkat Subramaniam', mPublicationDate=2014}
Book{mTitle='Clean Code', mAuthor='Robert C. Martin', mPublicationDate=2008}
Book{mTitle='Java Generics and Collections', mAuthor='Maurice Naftalin and Philip Wadler', mPublicationDate=2008}
Book{mTitle='Effective Java', mAuthor='Joshua Bloch', mPublicationDate=2008}
Book{mTitle='Pragmatic Unit Testing in Java 8 with JUnit', mAuthor='Jeff Langr', mPublicationDate=2015}
Book{mTitle='JavaFX Essentials', mAuthor='Mohamed Taman', mPublicationDate=2015}
Process finished with exit code 0
Why?
CamilleMarie Paugam
1,521 PointsCamilleMarie Paugam
1,521 PointsI also have the same question