Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial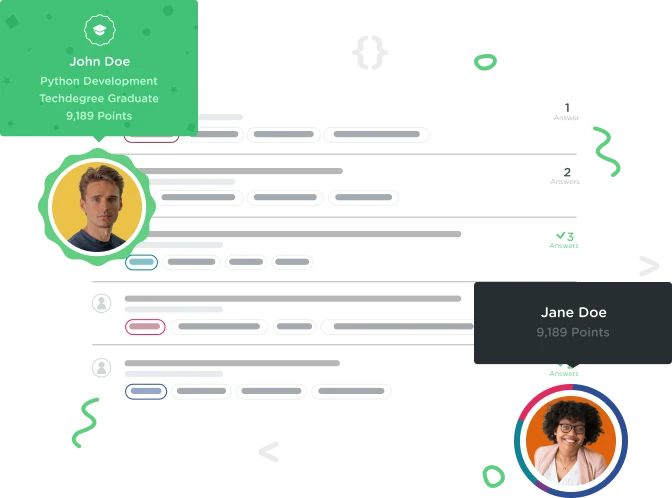
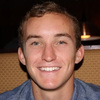
Stephen Clowes
4,512 PointsColor sliders not working
I'm having trouble debugging this block of code. The console isn't sending me any syntax errors. But, the #newColor span isn't changing colors with the sliders. Any help would be much appreciated!
// Set initial color
var color = $(".selected").css("background-color");
// When clicking on control list items
$(".controls li").click(function () {
// Deselect sibling elements
$(this).siblings().removeClass("selected");
// Select clicked element
$(this).addClass("selected");
// Cache current color
color = $(this).css("background-color");
});
// When new color is pressed
$("#revealColorSelect").click(function () {
// Show or hide colorSelect div
changeColor();
$("#colorSelect").toggle();
});
// Update the new color span
function changeColor() {
var r = $("#red").val();
var g = $("#green").val();
var b = $("#blue").val();
$("#newColor").css("background-color", "rgb(' + r + ', ' + g + ', ' + b + ')");
}
// When color sliders change
$("input[type=range]").on("input", changeColor);
1 Answer

Jason Anello
Courses Plus Student 94,610 PointsHi Stephen,
It doesn't look like you built up your rgb() string correctly.
You're trying to create a string that looks like rgb(255, 255, 255)
but using the values in r, g, and b
You have to build that up through string concatenation.
You used double quotes on the outside and single quotes inside which means your string is literally everything within the double quotes. The values for r, g, and b will not be substituted into that.
If you switch to all double quotes then it should work out. Let me know if you're not sure why.
Stephen Clowes
4,512 PointsStephen Clowes
4,512 PointsThank you so much, Jason! I got it work now. However, isn't it true in plain javascript you're supposed to use singe quotes if the statement is wrapped in double quotes?
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsThat is something different from what you're trying to do here.
If I had a string with a literal single quote in it, then I would want to wrap it in double quotes.
Example:
var sentence = "I'm going to the store.";
In this case, I want that single quote to be part of the string so I wrap it in double quotes. On the other hand, if I was going to have literal double quotes in my string then I would want to enclose the string with single quotes.
With this rgb() value we're simply trying to build up the proper rgb value that css requires. We don't want those single quotes and plus signs to be a part of the string. Think of it as we're adding together a bunch of small strings along with some variables.
It might help to understand it if you can see what the results are of your string and the corrected one.
Here's some console output:
In the first example you can see that the plus signs, single quotes, and variable names literally become part of the string.
In the second example, because the plus signs and variable names are outside of any string the plus signs will be accurately interpreted as string concatenation and the r, g, b will be interpreted as variable names and have their values substituted in. It's a bunch of short strings added together with some variables.