Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial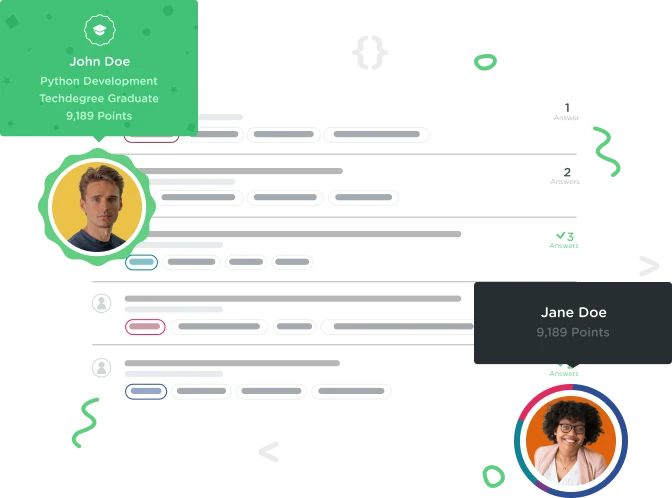

Micheal Corr
2,018 PointscolorLiteralRed not working - Swift 5 Xcode 11
Hi, Here is my code as instructed by the video:
enum ColorComponent {
case rgb(Float, Float, Float, Float)
case hsb(CGFloat, CGFloat, CGFloat, CGFloat)
func color() -> UIColor {
switch self {
case .rgb(let red, let green, let blue, let alpha): return UIColor(colorLiteralRed: red/255.0, green/255.0, blue/255.0, alpha/255.0)
case .hsb(let hue, let saturation, let brightness, let alpha): return UIColor(hue: hue/360.0, saturation: saturation/100.0, brightness: brightness/100.0, alpha: alpha)
}
}
}
ColorComponent.rgb(61.0, 120.0, 198.0, 1.0).color()
However, at colorLiteralRed it flags an error: "incorrect argument label"
I tried to take a look at the other initialisers available to me under UIColor and none had arguments that matched anything similar to UIColor(red: Float green: Float blue: Float alpha: Float)
The only thing i could find similar was UIColor(red: CGFloat, green: CGFloat, blue: CGFloat, alpha: CGFloat).
Will this achieve the same thing? My code now looks like this:
enum ColorComponent {
case rgb(CGFloat, CGFloat, CGFloat, CGFloat)
case hsb(CGFloat, CGFloat, CGFloat, CGFloat)
func color() -> UIColor {
switch self {
case .rgb(let red, let green, let blue, let alpha): return UIColor(red: red/255.0, green: green/255.0, blue: blue/255.0, alpha: alpha/255.0)
case .hsb(let hue, let saturation, let brightness, let alpha): return UIColor(hue: hue/360.0, saturation: saturation/100.0, brightness: brightness/100.0, alpha: alpha)
}
}
}
ColorComponent.rgb(61.0, 120.0, 198.0, 1.0).color()
Is this an acceptable workaround or am I on the complete wrong track? Im not sure if this is a compatibility issue with the versions or if I have just done something dumb somewhere to trigger the error.
Any help appreciated!
2 Answers

Victor Mercier
14,667 PointsHi, if you want to achieve the same thing with a newer version of xCode, just do the following:
enum ColorComponent{
case rgb(red:CGFloat,green:CGFloat,blue:CGFloat,alpha:CGFloat)
case hue(hue:CGFloat,saturation:CGFloat,brightness:CGFloat,alpha:CGFloat)
func getColor()->UIColor{
switch self {
case .rgb(let red,let green,let blue,let alpha):return UIColor.init(displayP3Red: red/255.0, green: green/255.0, blue: blue/255.0, alpha: alpha)
case .hue(let hue,let saturation,let brightness,let alpha):return UIColor.init(hue: hue/360.0, saturation: saturation/100.0, brightness: brightness/100.0, alpha: alpha)
}
}
}
If that helped you, please mark as best answer to indicate other students your issue is resolved!

Victor Mercier
14,667 PointsJust to say that your code should probably be working also!
Micheal Corr
2,018 PointsMicheal Corr
2,018 PointsHi Victor, Thanks for the quick reply - this seems to work for me!
Can you provide any further explanation on the difference between the (displayP3Red, green, blue, alpha) initialiser and the one that just takes red, green, blue, alpha in my example?