Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial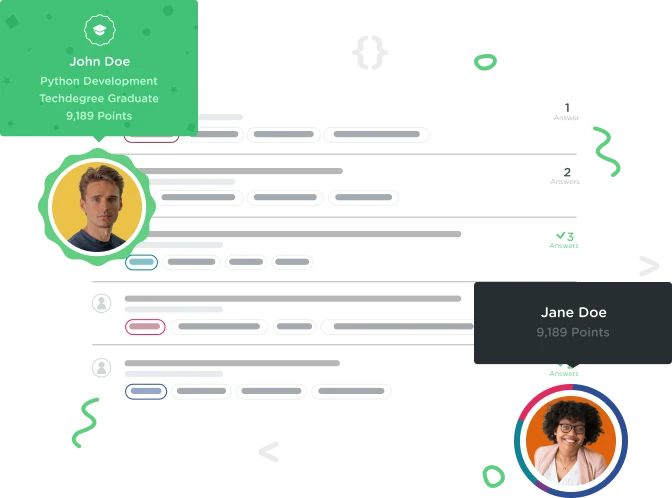

David Drysdale
10,815 PointsColour issues: when I change colours, the existing lines change to the new colour.
Not sure what I've done wrong.
If I draw a line, then select another colour, and then start drawing a new line, the existing line changes colour. The colour change happens when I mouse down after selecting the new colour.
Here is my code:
//Problem: No user interaction causes no change to application
//Solution: When user interacts cause changes appropriately
var color = $(".selected").css("background-color");
var $canvas = $("canvas");
var context = $canvas[0].getContext("2d");
var lastEvent;
var mouseDown = false;
//When clicking on control list items
$(".controls").on("click", "li", function(){
//Deselect sibling elements
$(this).siblings().removeClass("selected");
//Select clicked elements
$(this).addClass("selected");
//cache current color
color = $(this).css("background-color");
});
//When new color is pressed
$("#revealColorSelect").click(function(){
// Show or hide the color select
changeColor();
$("#colorSelect").toggle();
});
//update the new color span
function changeColor() {
var r = $("#red").val();
var g = $("#green").val();
var b = $("#blue").val();
$("#newColor").css("background-color", "rgb(" + r + ", " + g + ", " + b + ")");
}
//When color sliders change
$("input[type=range]").on("input", changeColor);
//When "add color" is pressed
$("#addNewColor").click(function(){
//Append the color to the controls ul
var $newColor = $("<li></li>");
$newColor.css("background-color", $("#newColor").css("background-color"));
$(".controls ul").append($newColor);
//Select the new color
$newColor.click();
});
//On mouse events on the canvas
$canvas.mousedown(function(e){
lastEvent = e;
mouseDown = true;
}).mousemove(function(e){
//draw lines
if(mouseDown) {
context.moveTo(lastEvent.offsetX, lastEvent.offsetY);
context.lineTo(e.offsetX, e.offsetY);
context.strokeStyle = color;
context.stroke();
lastEvent = e;
}
}).mouseup(function(){
mouseDown = false;
}).mouseleave(function(){
$canvas.mouseup();
});
2 Answers

David Drysdale
10,815 PointsThanks!
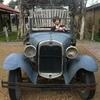
Brendan Moran
14,052 PointsSo interesting! I would like to understand why the lack of the .beginpath() causes the existing lines to change color. i would just expect the drawing not to work!

Ulan Assanoff
9,922 PointsThe beginPath() method begins a path, or resets the current path. http://www.w3schools.com/tags/tryit.asp?filename=tryhtml5_canvas_beginpath you do not reset the previous color without The beginPath() method .
Vitaliy Bogdanov
7,612 PointsVitaliy Bogdanov
7,612 PointsHi David!
In section with canvas drawing you need add "context.beginPath();" method.
Like this: