Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial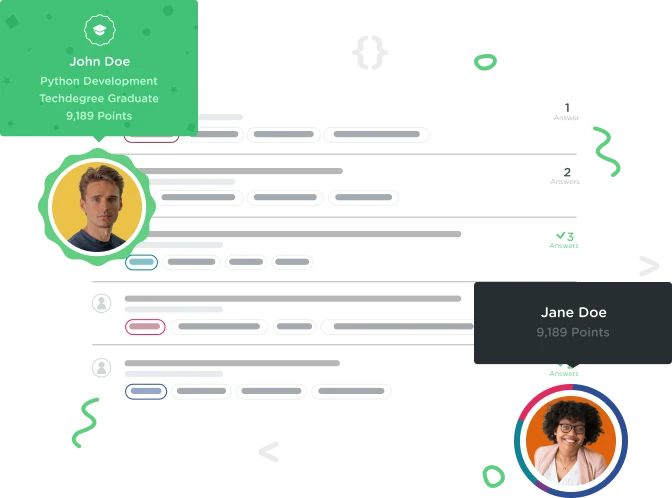

Piotr Mozgawa
5,937 Pointscombiner Bummer: Didn't get the expected result, while in workspace I get it.
What is wrong with my code? I get the exact result in workspace but the task says it is wrong.
;;;python def combiner(*args): string = "" sum = 0 for item in args: if isinstance(item, str): string += item if isinstance(item, (int, float)): sum += item return string+str(sum) ;;;
def combiner(*args):
string = ""
sum = 0
for item in args:
if isinstance(item, str):
string += item
if isinstance(item, (int, float)):
sum += item
return string+str(sum)
1 Answer
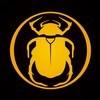
rydavim
18,813 PointsDon't worry, you've got the right idea. It looks like it's just a really simple problem with how *args works.
*args is a really cool shorthand that lets you pass in a variable number of arguments, which is really useful when you're not sure how many you'll need. However, that means that what you're passing in with your code is a collection of arguments. Since the challenge is only giving you a single argument, this would be a collection of only 1 item. The problem is that you're then iterating over the items in args.
For example, let's assume the argument you're given is ["apple", 5.2, "dog", 8]
.
def combiner(*args): # combiner(["apple", 5.2, "dog", 8])
string = ""
sum = 0
for item in args: # for item in [["apple", 5.2, "dog", 8]] (see that the whole collection is a single item)
if isinstance(item, str): # a collection is not a str, this never runs
string += item
if isinstance(item, (int, float)): # a collection is not an int or float, still doesn't run
sum += item
return string+str(sum) # returns an empty str and 0
Try just passing a single argument combiner(args)
. This would be a quick and dirty way to fix the issue.
I'm a little bit surprised that it's coming up normally when you try it in workspaces. You're getting the expected result with that code and the given input of ["apple", 5.2, "dog", 8]
?
In any case, hopefully that all makes sense, but let me know if you have any questions or still feel stuck. Good luck, and happy coding!