Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial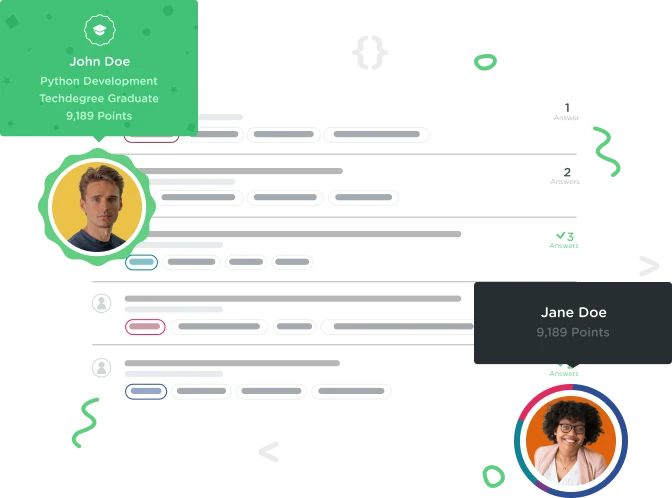

Xavi Guasch
10,882 PointsCombining filter() and map(), challenge doubt
Can't seem to locate my error. This is my proposed solution:
const todos = [
{
todo: 'Buy apples',
done: false
},
{
todo: 'Wash car',
done: true
},
{
todo: 'Write web app',
done: false
},
{
todo: 'Read MDN page on JavaScript arrays',
done: true
},
{
todo: 'Call mom',
done: false
}
];
let unfinishedTasks;
// unfinishedTasks should be: ["Buy apples", "Write web app", "Call mom"]
// Write your code below
unfinishedTasks = todos
.filter(todo => todo.done = false)
.map(todo => {(todo)});
console.log(unfinishedTasks);
6 Answers

Manish Giri
16,266 PointsThis line looks wrong - .filter(todo => todo.done = false)
.
I'm assuming you want to filter todo
items whose done
field is false
. You'll need to use ==
or ===
to compare the two values then. Using =
just assigns false
to todo.done
, which doesn't make sense in case of a .filter()
.
A concise approach would be - .filter(todo => !todo.done)
.

Xavi Guasch
10,882 PointsSince you need to pull the todo field from the object, try .map(e => e.todo).
This works, but I don't really understand the use of the "e" here... Could you please elaborate?

Manish Giri
16,266 PointsWhen you iterate over an array with a for loop, you pick a counter variable, like -
for(var i = 0; i < arr.length; i++) {
// you can now refer to each array item by arr[i]
}
It's the same thing with functions like .map()
, .filter()
. You create a variable, which would be the current array item you're iterating upon. Generally, e
is considered short for element
, so people use e
. You can use any name you like, ex - .map(currentItem => currentItem.todo);
.
Hope this helps.
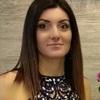
Diana Ci
18,672 PointsunfinishedTasks = todos
.filter(todos => todos.done === false)
.map(todos => todos.todo);
console.log(unfinishedTasks);

Xavi Guasch
10,882 PointsThanks, Manish. But there must be another error, as it still doesn't work.

Manish Giri
16,266 PointsThe .map()
line looks wrong to me - .map(todo => {(todo)})
, unless you were trying to use Object Destructuring.
Since you need to pull the todo
field from the object, try .map(e => e.todo)
.

Seth Kroger
56,414 PointsA { immediately after the arrow is considered the start of a function block and you'd need an explicit return statement to return anything. If you want to return an object literal in the shorthand syntax you need to wrap it in parentheses on the outside: ({ todo })
It looks like you got it reversed for what you intended. Not the correct answer for the challenge though.

Xavi Guasch
10,882 PointsGot it! Thanks again, Manish.

Andreas Vilhelmsson
1,831 Pointsi think this should work
let unfinish = todos.filter(function(todoo){ return todoo.done === false; });
console.log(unfinish);
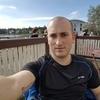
Vladimir Chagorovski
Full Stack JavaScript Techdegree Student 12,965 PointsIs this code okay for this exercise
const userNames = ['Samir', 'Angela', 'Beatrice', 'Shaniqua', 'Marvin', 'Sean'];
// Result: [{name: 'Samir'}, {name: 'Shaniqua'}, {name:'Sean'}];
const names = userNames
.filter(name => name.includes('S'))
.map(name =>`{name: ${name}}`);
console.log(names);