Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial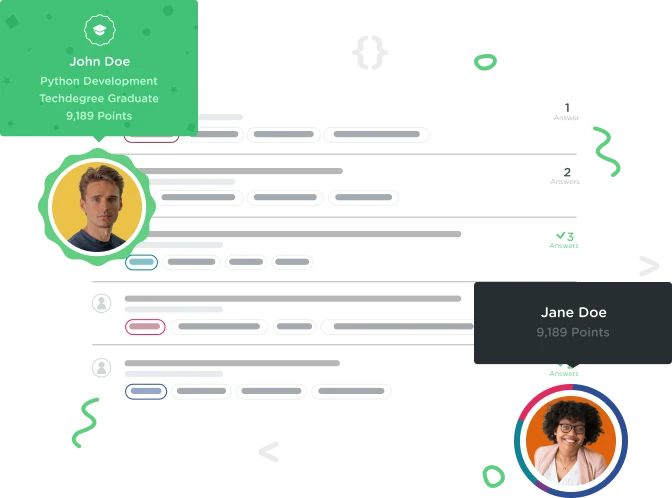

Aakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 Pointscombining filter() and reduce()
Why didn't this worked -- Steven Parker
const products = [{
name: 'hard drive',
price: 59.99
},
{
name: 'lighbulbs',
price: 2.59
},
{
name: 'parker pens',
price: 1.49
},
{
name: 'paper towels',
price: 6.99
},
{
name: 'flatscreen monitor',
price: 159.99
},
{
name: 'cable ties',
price: 19.99
},
{
name: 'ballpoint pens',
price: 2.49
}
];
const totalPrice = products.filter(product => product.price > 10).reduce((total, product) => {
return (total.price + product.price);
},{price: 0}).toFixed(2);
console.log(totalPrice);
I have used return total.price + product.price
. This is not working.
If i am using total.price
, it should extract the price and then add it to the product.price
but it is not happening. I have also set an initial object with price
property to 0
3 Answers
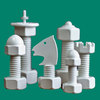
Steven Parker
231,275 Points I was alerted by your tag. But there are many students willing to help; so give the community a chance to respond when you post a question before tagging anyone specifically.
The return from the callback given to "reduce" must be the same type as the accumulator and the initial value, which in this case is an object with a "price" property:
return { price: total.price + product.price };
// ^^^^^^^^ ^
Then, since that is also the return type of the "reduce", you will need to access the "price" property to get the total:
/*...*/).price.toFixed(2);
// ^^^^^^
But a simpler approach would be to make the accumulator and initial value just numbers:
/*...*/.reduce((total, product) => total + product.price, 0).toFixed(2);

Aakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsI am loving the explanation but still not getting 100% . Will you elaborate a little bit more . Please ?
What does this will return : return (total.price + product.price);
?
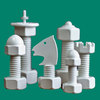
Steven Parker
231,275 PointsThat line from the original code returns an ordinary number, but that's not compatible with the accumulator being an object. So it must return an object also (first example), or the entire thing must be changed to work with plain numbers (second example).

Aakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 Pointsah Thanks a lot :)
Aakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsAakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsSorry for tagging you , but I was not getting the answer of this doubt since morning , I posted in slack forum too , but no one replied and this doubt was eating me. So , i reached to you .
In your explanation , What does this mean :
/*...*/.reduce(/*...*/)
?Steven Parker
231,275 PointsSteven Parker
231,275 PointsThose comments are just placeholders for parts of the code I left out to shorten the example.