Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial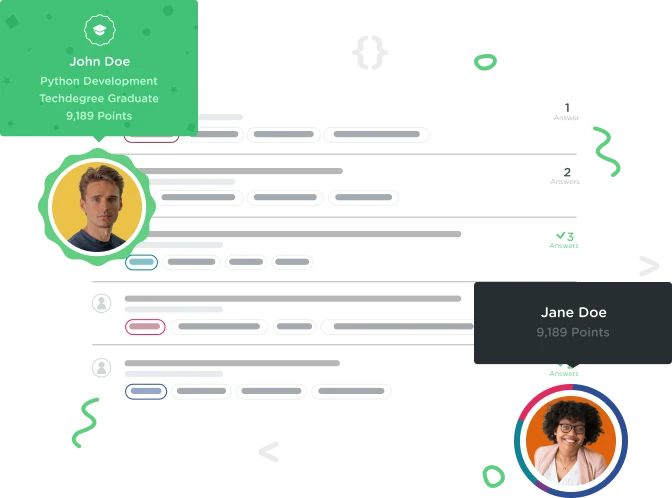

Andrew Mlamba
15,642 PointsCombo
Need some help here. The grader is not passing a helpfull message for me to understand and rectify my code.
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo(iterable_1, iterable_2):
output = []
for index in range(len(iterable_1)):
t = iterable_1[index], iterable_2[index]
output.append(t)
return output
3 Answers

Umesh Ravji
42,386 Points[Edit] Since the questions 'answer acceptance engine' has been changed, please see this thread: https://teamtreehouse.com/community/works-in-ide-not-in-challenge
Your answer works fine for the example provided. However, it won't work for dictionaries and sets because it won't work for collections that are not indexed based. You have to use iterators.
I doubt my way of doing it was the best, but I hope it should be enough to point you in the right direction.
def combo(a, b):
iter_a = iter(a)
iter_b = iter(b)
results = []
# use iterators and generate results here
return results

Umesh Ravji
42,386 PointsMaybe it's a bug, but I'm not sure :)
Bummer! Example output: `combo('abc', 'def')` => <zip object at 0x7ffb5d63fac8>
However, this works fine:
def combo(a, b):
return list(zip(a, b))
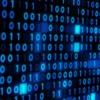
Alexander Davison
65,469 PointsOops XD
I always forget to add list()
. Thanks for reminding me!
~Alex
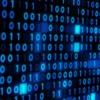
Alexander Davison
65,469 PointsAdding on to Umesh's answer
In fact, there's an even easier way of doing this. To get rid of all this hassle, you can just use a handy-dandy function called
zip
.
Try this:
def combo(a, b):
return list(zip(a, b))
You might be wondering something like "What the?... Why does Python have this useless function that I'll never use again?", however, later you'll find out that you can use zip
in for
loops, and, volia! You get to hold on to two variables. Wait until you see a future video and you'll get it
Finally, we convert zip
into a list so that it will return what you'd expect and not a zip
object. (Thanks Umesh for pointing that out I would've forgetten lol)
I hope this post helps as well :)
Good luck!
~Alex
EDITED: Added list()
around zip()