Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial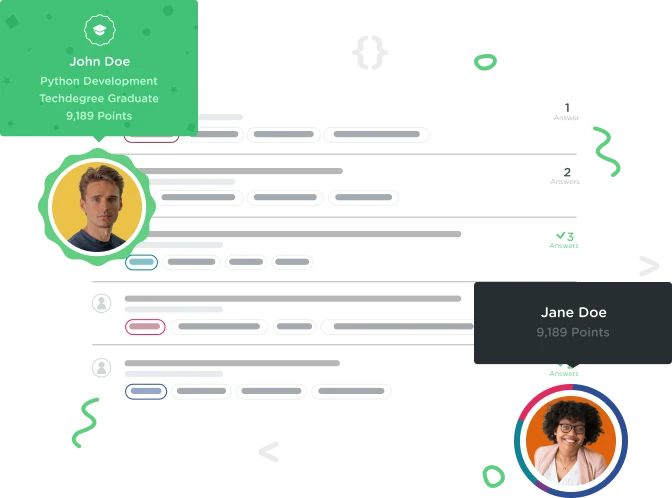

Dustin Jasmin
3,156 PointsCombo
Can someone please help me out with the combo challenge in python basics, I've tried but I don't understand it at all. Can someone please assist me.

Dustin Jasmin
3,156 Points2 Answers

matth89
17,826 PointsStart with a new empty list inside your function, then use a for loop to iterate through the arguments (remember, the challenge says both arguments will be the same length, so iterating over either one works), then on each pass in the loop append a single tuple with the current index's value from both argument one, and argument two to your previously empty list. Last, return your created list. I hope this helps!
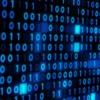
Alexander Davison
65,469 Points Nice answer, but it's a little confusing to understand your answer.

matth89
17,826 PointsI could see that. I'm definitely no teacher. Just sharing what I can.
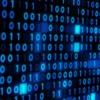
Alexander Davison
65,469 Points You're on your way!
(If you want to be a teacher, I mean.)
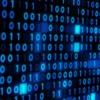
Alexander Davison
65,469 PointsRead the problem more carefully:
Create a function named combo that takes two ordered iterables. These could be tuples, lists, strings, whatever.
Your function should return a list of tuples. Each tuple should hold the first item in each iterable, then the second set, then the third, and so on. Assume the iterables will be the same length.
Check the code below for an example.
- The first line ("Create a function named combo ...") says that your function takes two iterables. An iterable can be a string, a tuple, a list, etc.
- The second line says that your function should return a list of tuples. Each tuple in the list holds the first value in the first iterable and the first value in the second iterable, the second value in the first iterable and the second value in the second iterable, etc. For example:
combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
As you can see, the first tuple in the returned list contained the first values of each of the iterables, the second tuple hold the values of the second values in each iterable, etc.
Here's a working solution:
def combo(iter1, iter2):
result = []
for i in range(len(iter1)):
result.append((iter1[i], iter2[i]))
return result
The solution is simple:
- We make a function that takes two arguments:
iter1
anditer2
. - We set up the
result
list. - We make a for loop. The variable
i
keeps track of the index. - We extract the values from the two iterables using the index then append it to
result
. - We return
result
.
Does this clear things up?
I hope this helps!
Happy coding! ~Alex

Dustin Jasmin
3,156 PointsI'm still a little confused because since you made the i in the for loop for range(len(iter1)) won't that I be for every number not for every value, and so if that's so when you append (iter1[i], iter2[I]) won't those be two numbers?
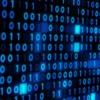
Alexander Davison
65,469 PointsNo, the extra parentheses around (iter1[i], iter2[i])
make it a tuple.
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsI cannot find a challenge named "combo" in Python Basics. Can you link to the problem? I can then help you