Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial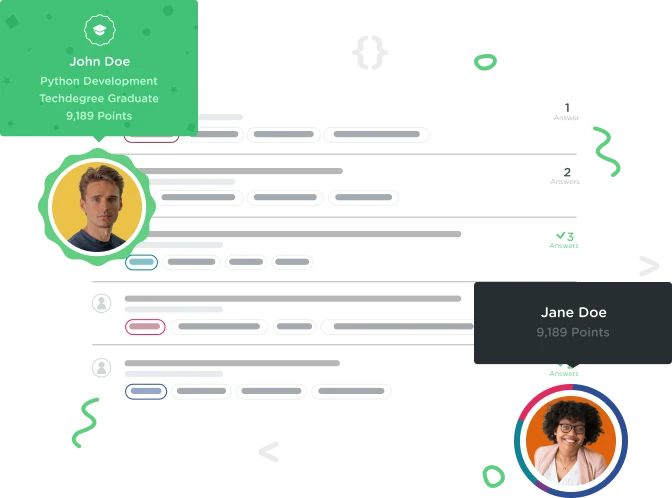

Frank Sherlotti
Courses Plus Student 1,952 PointsCombo challenge: Please help.
QUESTION -- Create a function named combo() that takes two iterables and returns a list of tuples. Each tuple should hold the first item in each list, then the second set, then the third, and so on. Assume the iterables will be the same length. I've been messing with this for a few days now and I've made no headway and it's infuriating me. I was wondering if someone could just give me the answer at this point, or just give me obvious hints to what the answer is. Really do appreciate anyone who does decide to help, thanks!!
combo(['swallow', 'snake', 'parrot'], 'abc')
Output:
[('swallow', 'a'), ('snake', 'b'), ('parrot', 'c')]
If you use list.append(), you'll want to pass it a tuple of new values.
Using enumerate() here can save you a variable or two.
def combo(first, second):
empty = ()
for index, value in enumerate(first):
empty = first
empty.append(second)
return empty
2 Answers
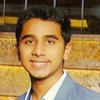
Anish Walawalkar
8,534 PointsYou can use the zip(first, second). this will return a list of tuples. that will solve your problem
return zip(first, second)
this will also fix your problem:
def combo(item1, item2):
x = []
for index, item in enumerate(item2):
x.append((item1[index], item))
return x
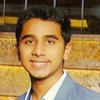
Anish Walawalkar
8,534 PointsSay you have a list of names:
names = ['Frank', 'John', 'Anish']
so when you do:
for index, value in enumerate(names):
enumerate returns a tuple of (index, value) for each element of the list. for example when the for loop runs the first time, the enumerate(names) function returns (0, 'Frank') which gets stored as index=0 and value = 'Frank'
so finally to answer your first question: NO there is not difference b/w for index, value and for index, item
Now for your second question: you do not need to run a for loop. zip(first, second) is an inbuilt python function that handles what the for loop is doing internally. So if you were to use the zip(first, second) your function should look like this:
def combof(first, second):
return zip(first, second)
Hope this answers your question. Anish

Frank Sherlotti
Courses Plus Student 1,952 PointsReally appreciate the help ! THANKS!
Frank Sherlotti
Courses Plus Student 1,952 PointsFrank Sherlotti
Courses Plus Student 1,952 PointsWhich would be the correct way to do it so I know for future reference. And also THANK YOU VERY MUCH! You have no idea how much that helps me out I really do appreciate it.
Anish Walawalkar
8,534 PointsAnish Walawalkar
8,534 PointsWell its always better to use an inbuilt language feature. So using the zip() function is a better way of doing it.
Frank Sherlotti
Courses Plus Student 1,952 PointsFrank Sherlotti
Courses Plus Student 1,952 PointsWhat's the difference between for index, item and for index, value? And would the return zip(first, second) way look like this? I don't see how this zip method works.