Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial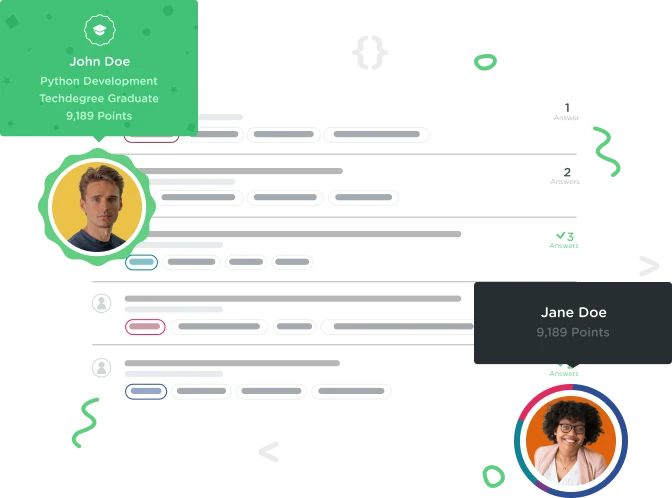

Tilak Muruduru Divakar
6,459 Points'combo' function challenge
I have written a function which works just fine. But I have a feeling that this can be written in a better way. Since 'tuples' are immutable, I couldn't think of a different way to create(I need to take the second item of the tuple from a different iterable) the tuple. Could someone please suggest some improvements? Is there a way to iterate over two iterables in the same loop like: for a, b in iterable1, iterable2?
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo(iter1,iter2):
lists_of_lists = []
for item in iter1:
lists_of_lists.append(list(item))
index = 0
for item in iter2:
lists_of_lists[index].append(item)
index += 1
list_of_tuples = []
for combo_list in lists_of_lists:
list_of_tuples.append(tuple(combo_list))
return list_of_tuples
2 Answers

Pete P
7,613 PointsSomething that may help is the enumerate() function. As long as your iterables are the same length, you could do something like this:
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo(iter1, iter2):
list_of_tuples = []
for index, item in enumerate(iter1):
a_tuple = item, iter2[index]
list_of_tuples.append(a_tuple)
return list_of_tuples
Though, I'm curious to see other's ideas as well. Possibly using zip()?
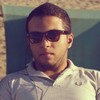
Noor Elharty
2,630 Pointsi have the same question as Dustin Jasmin ? can some one clarify what index do ?
Pete P
7,613 PointsPete P
7,613 PointsI had to learn about the zip() function a bit. But, seems you could also do this:
or even shorter
Dustin Jasmin
3,156 PointsDustin Jasmin
3,156 PointsHey, I'm currently on this challenge and my question is what does [index] do in iter2[index]?