Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial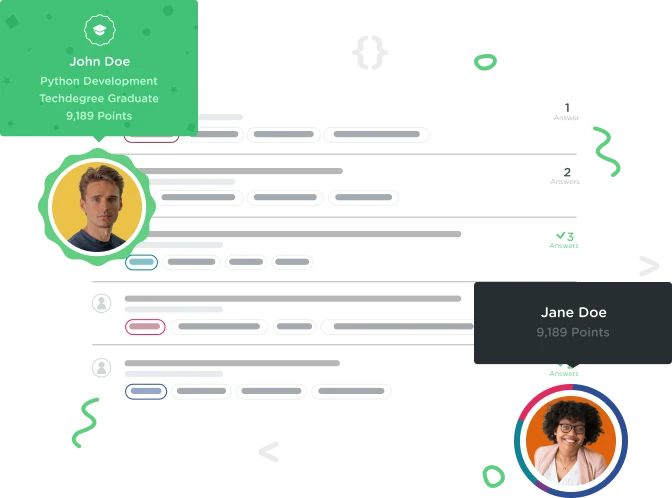

Virginia Hickman
1,862 PointsCombo Tuple Challenge Task
I can't figure out why it doesn't return it into a list. I can't put the return statement anywhere else to make it loop through all the variables.
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo(arg1,arg2):
for args1 in arg1:
for args2 in arg2:
return {(args1,args2)}
1 Answer
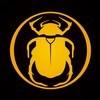
rydavim
18,814 PointsOkay, so let's run through what's happening with your code.
# combo([1, 2, 3], 'abc') - We'll use this as example input.
def combo(arg1,arg2): # Yup.
for args1 in arg1: # Starting this iteration, you've now 'got' the 1.
# But then you go into the next for loop.
for args2 in arg2: # This loop would continue, without exiting.
return {(args1,args2)} # Except that you return here, exiting out of the entire function.
# So what you end up with out here, is {(1, 'a')} only.
You'll need to iterate through the two inputs together, in order to ensure you're not getting all of the things in one list before going back to the first. Right now even if you didn't return
early, you'd end up with repeating arguments.
def combo(arg1,arg2):
for args1 in arg1:
for args2 in arg2:
print({args1, args2})
#return {(args1,args2)}
# You'll get output something like...
# {1, 'a'} {1, 'b'} ... {3, 'b'} {3, 'c'}
There are several different ways to solve this, but you can try using the pseudo-code below to work on a solution.
# def combo(arg1,arg2):
# Create an empty tuple list variable to hold the created tuples.
# Use a for loop to iterate through the inputs. Try using enumerate() here.
# Create a new tuple to hold your current item, and the [index] of the other input. Gotta' do them together.
# Now you can append() this created tuple to the tuple list you have outside the loop.
# Once you've got all your tuples appended, return the tuple list.
Hopefully that helps you get started, but please let me know if you have any questions or if you'd like to walk through a potential solution. Happy coding!
Virginia Hickman
1,862 PointsVirginia Hickman
1,862 PointsGot it! Thank you!