Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial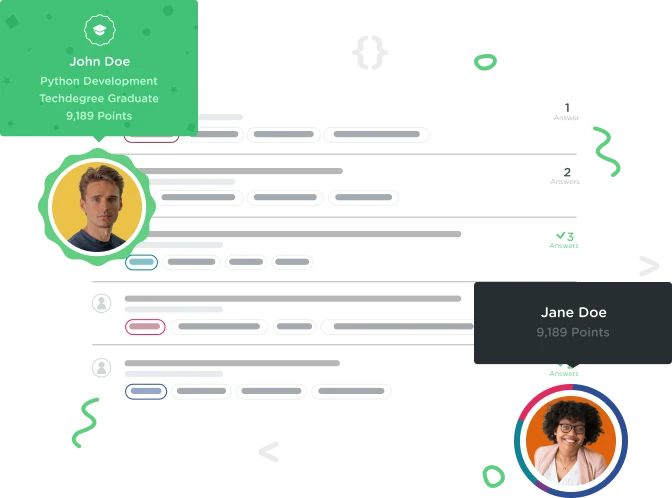

Tomme Lang
1,118 Pointscombo.py
Trying to solve this using enumerate. Not sure what I am missing
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo(thing1, thing2):
return list(enumerate(thing1, thing2))
1 Answer

Jeffrey James
2,636 PointsPretty common to have to combine 2 iterables pairwise, a common pattern is this:
>>> list(zip([1, 2, 3], 'abc'))
[(1, 'a'), (2, 'b'), (3, 'c')]
Another common pattern is creating a dictionary from 2 iterables, where one will contain your keys and the other the corresponding values. Make sure that in this case, each key will be unique:
>>> dict(zip([1, 2, 3], 'abc'))
{1: 'a', 2: 'b', 3: 'c'}
Enumerate is typically used for when you're iterating over a list or set or dict items and you need to keep track of the index or position in the loop. Here's a trippy example of enumerate, within a list comprehension
>>> [(idx**5, a, b) for idx, (a, b) in enumerate(list(zip([1, 2, 3], 'abc')))]
[(0, 1, 'a'), (1, 2, 'b'), (32, 3, 'c')]
Above, you can see that enumerate throws off an index, which I'm calling "idx", but could be called anything. See what happens when (a,b) is called without the parens if you try and grok it.
One last note, the idea of an object being an iterable is important in python. If something does not have an iter method implemented on its class (like a list or set or string), then you cannot loop over it. But note how in the below example, 'abc' was in a list of strings, rather than a single string and the result is still the same. That's because if you iterate over a string, or a list of strings (letters), it will yield the same thing (letters).
>>> list(zip([1, 2, 3], ['a', 'b', 'c']))
[(1, 'a'), (2, 'b'), (3, 'c')]
More food for thought
>>> list(zip([1, 2, 3], "".join(['a', 'b', 'c'])))
[(1, 'a'), (2, 'b'), (3, 'c')]

Christian Lamoureux
Full Stack JavaScript Techdegree Student 22,669 PointsIf you use zip the challenge no joke rejects it and tells you I know zip() exists and to figure it out yourself.
Tomme Lang
1,118 PointsTomme Lang
1,118 PointsHere is the question
Alright, this one can be tricky but I'm sure you can do it. Create a function named combo that takes two ordered iterables. These could be tuples, lists, strings, whatever. Your function should return a list of tuples. Each tuple should hold the first item in each iterable, then the second set, then the third, and so on. Assume the iterables will be the same length. Check the code below for an example.