Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial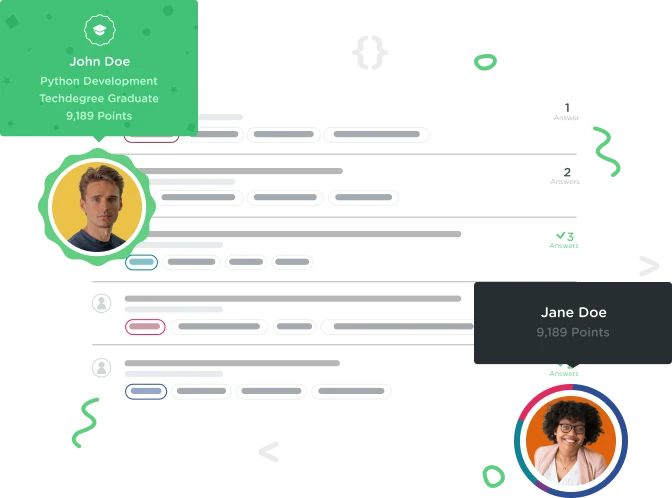
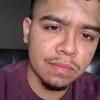
David Garcia
Python Development Techdegree Graduate 11,254 Pointscombo.py
I need to go more into depth with tuples and dicts.. anyways I need help with this one have any hints to what I should do, I understand the concept but not the input im supposed to give..
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo(combos):
combos = ([1,2,3],"abc")
enumerate(combos)
return combos
2 Answers
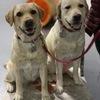
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi David,
The question text is not perfectly clear about what your function signature should look like (is it a single argument containing two iterables, or is it two arguments, containing an iterable each?). Your code indicates that you've read the text as the former. This is perfectly reasonable but the first line of the example code provided shows that the question really wants the latter. Thus your function definition should take exactly two arguments.
In the first line of your function, you are replacing the values that are passed in to your function with a literal: [1, 2, 3], 'abc'
which means that your function will always return exactly the same value no matter what is passed into it. The question specifies:
Create a function named combo that takes two ordered iterables. These could be tuples, lists, strings, whatever. Your function should return a list of tuples. Each tuple should hold the first item in each iterable, then the second set, then the third, and so on. Assume the iterables will be the same length.
Therefore the question wants you to transform some arbitrary values that are passed in as arguments into a single
output that still contains the same underlying values.
So, if your function were passed [1, 2, 3]
and 'abc'
you would be expected to return:
[(1, 'a'), (2, 'b'), (3, 'c')]
But if it were passed 'hello'
and ('foo', 'bar', 'baz', 'dog', 'cat')
you would be expected to return:
[('h', 'foo'), ('e', 'bar'), ('l', 'baz'), ('l', 'dog'), ('o', 'cat')]
Hopefully that clears up what the question is asking for. If you are still struggling with the implementation, let me know and I'll give you some more hints.
Cheers
Alex
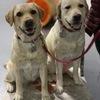
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi David,
Without proper formatting, I can't see exactly what your code is doing. I'm going to assume that you meant for you code to look something like this:
def combo(tuple, list):
tuple = ('a','b','c')
list = ['d', 'e', 'f']
combos = tuple, list
combos(enumerate(tuple,list))
for index, letter in enumerate(combos)
return combos
combo()
Here are some of the issues:
- You've given your arguments names of
tuple
andlist
. However, this can be very misleading, since the question clearly tells you that your function will be passed "two ordered iterables. These could be tuples, lists, strings, whatever." So you might get passed a tuple, then a list, or you might get two strings, or a list and a string, etc. You need to be able to handle any combination of two ordered iterables. That said, Python doesn't care if you use misleading variable names, but it is likely that it will prompt you to write incorrect code, and anyone reading your code will be confused. - Inside your function you immediately replace whatever values were passed into your function with the literal values of
('a', 'b', 'c')
and['d', 'e', 'f']
, respectively. This means that if the question called your function like this:
combo([1, 2, 3], 'abc')
(which is quite likely, considering it is exactly the example it provides you in the question), the very first thing your combo function will do is throw away the [1, 2, 3]
and replace it with ('a', 'b', 'c')
and throw away the 'abc' and replace it with ['d', 'e', 'f']
. The question tells you in the example that it expects you to output [(1, 'a'), (2, 'b'), (3, 'c')]
(i.e., a transformation of the input values) for that set of inputs. You won't be able to do that if the first thing you do is throw away the inputs.
- This line makes no sense:
combos(enumerate(tuple,list))
. You can't call enumerate on a tuple then a list in a single call. Enumerate takes one or two arguments, the first is always the iterable you want to enumerate over, and the second (optional) argument is a single integer which defines which index in the iterable you want to start with. Also, combos was defined in the previous line as a tuple (containingtuple
andlist
). Tuples aren't callable so you can't use the parentheses. - In the
for index, letter...
line, you don't finish the line with a colon. This causes a syntax error. - In the final line you are calling your function, but the question doesn't tell you to call your function. The question grader will call your function (probably many times, and with a variety of different arguments). Don't write code that isn't asked for by the question.
- Even if the question did ask you to call your function, your function call is invalid. You've defined combo as a function that must take 2 arguments (
tuple
andlist
), but you've called it with zero arguments. A valid call would look something like:
combo(('a', 'b', 'c'), [1, 2, 3])
I suggest you re-read the exact wording of the question. It tells you what kind of inputs you should expect for your function, and what sort of outputs your function should provide for a particular set of inputs.
It also gives you some hints about what techniques you should apply to solve the question, such as:
Assume the iterables will be the same length
If you know you can rely on the iterables being the same length, what common function can you use to get that length?
Once you have a particular length, is there a loop construct that you could use to repeat that many times, with an index you could use to access the equivalent value in each of your inputs?
Hopefully these hints will point you in the right direction. If you need any more hints, let me know.
Cheers
Alex
David Garcia
Python Development Techdegree Graduate 11,254 PointsDavid Garcia
Python Development Techdegree Graduate 11,254 PointsAlex Koumparos still having trouble with is one heres what i did, i know what its talking about but my execution is poor...
def combo(tuple, list): tuple = ('a','b','c') list = ['d', 'e', 'f'] combos = tuple, list combos(enumerate(tuple,list)) for index, letter in enumerate(combos) return combos
combo()