Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial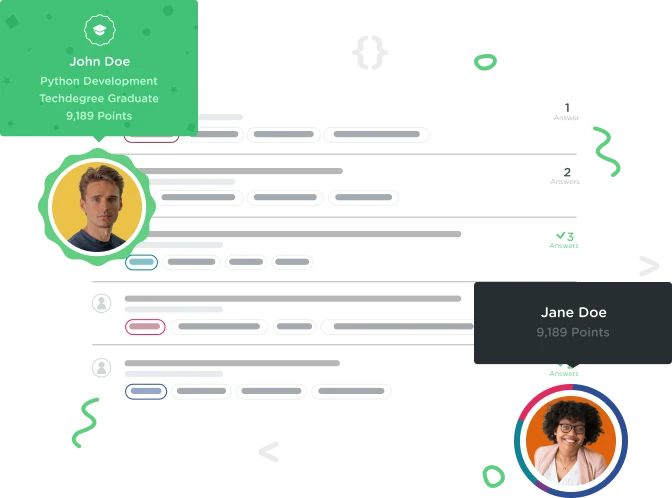

tangtai chen
4,844 Pointscompare the length of values for two keys in a dictionary.
The task ask me to compare the length of values for different keys in a single dictionary, but i couldn't come up with a way to do it, i was thinking using for loops, but for loops override values each loop so it won't able to compare the length of each value. can someone help?
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(teacher_course):
return len(teacher_course)
def num_courses(teacher_course):
# number_of_courses = sum(map(len, teacher_course.values()))
return sum(map(len, teacher_course.values()))
def courses(teacher_course):
list = []
for courses in teacher_course.values():
for each_course in courses:
list.append(each_course)
return list
4 Answers
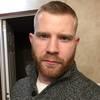
Christian Rowden
2,278 PointsHey there. I've posted my test code below, I was having problems with this task and so I started a test environment and this is what I evened up with that worked. I also couldn't find a way to find the maximum length of a value and then correlate that to a specific key... It had me stumped for quite a while. What you can do though is loop through a dictionaries KEYS and correlate those to different sets of VALUES. With the code below and a bit of testing I think that you should be able to come to a solution. =D
Cheers.
""" sample_dict = { 'James': ['Course', 'Table', 'Bird'], 'Kelly': ['Tiller', 'Table'], 'Jill': ['Jumps'], 'Chris': ['Jumps', 'Table', 'Bird'], 'Davis': ['Tiller', 'Hello', 'Brat'] }
max_val = 0 teacher = ""
for k in sample_dict.keys(): print(len(sample_dict[k])) if max_val < len(sample_dict[k]): max_val = len(sample_dict[k]) teacher = k print('\n') print(max_val) print(teacher)
"""
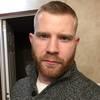
Christian Rowden
2,278 PointsHello,
I wanted to let you know that your code appears correct to my eyes. I have the exact same code and it works.
def courses(arg): single_list = [] for v in arg.values(): for items in v: single_list.append(items) return single_list
It works for me.
I was thinking however that maybe because your list is named list? I would toss it into my python on my desktop and run it with a made up list to see what kind of error or output you're receiving.
Cheers and happy coding.

tangtai chen
4,844 Pointsha yes, task3 works for me, sorry about the confusion, i only post codes for task 1-3, i was not able to write a working code for task4, hence i didn't post any for that matter. if you can give me a hint about task4 that would be great!
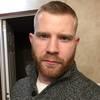
Christian Rowden
2,278 PointsAh I see what you mean.
Ok, so you're already on the right track. You can use a for loop to compare the sizes of the value lists by storing one in a variable and then comparing each new iteration against THAT variable.
max_var = 0 for x in in list: if max_var < x: x = max_var
print max_var
This is an example that should get you started in the right direction I think.
Cheers

tangtai chen
4,844 Pointsthanks for the reply, i used your method try to figure out a way to do it, but it could not return the right result, here is my code:
def most_courses(teacher_course): for courses in teacher_course.values(): max_var = 0 if len(courses) > max_var: max_var = len(courses) print(max_var)
i think it would try to compare the length of the courses and return the maximum value, but what it did is print random values of the numbers of the course. i don't know where went wrong. and one more thing, once i got the maximum length of the courses how do i call out the related teachers' name?
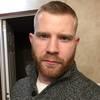
Christian Rowden
2,278 PointsSo, you're really close but with the .values() you're looking at and iterating through the values. I posted my complete code earlier but it looks as if it has been deleted... What you must do is iterate through dict[k].
If you run a print(dict[k] for k in dict) it will print the values of each key. This was the AHA! moment for me. If you still can't figure it out, shoot me a PM and I'll provide my code and walk your through it. This one had me stumped for a while.

tangtai chen
4,844 Pointsdef most_courses(sample_dict): max_val = 0 teacher = "" courses_list = {} for k in sample_dict.keys(): # print(len(sample_dict[k])) if max_val < len(sample_dict[k]): max_val = len(sample_dict[k]) teacher = k courses_list = sample_dict[k] # print(max_val) print(teacher) print(courses_list)
so i followed your code and got a working code, only a final step left to do here i think is to return the output in the form of a dictionary can you help me out here?
tangtai chen
4,844 Pointstangtai chen
4,844 Pointsmany thanks for the help!