Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial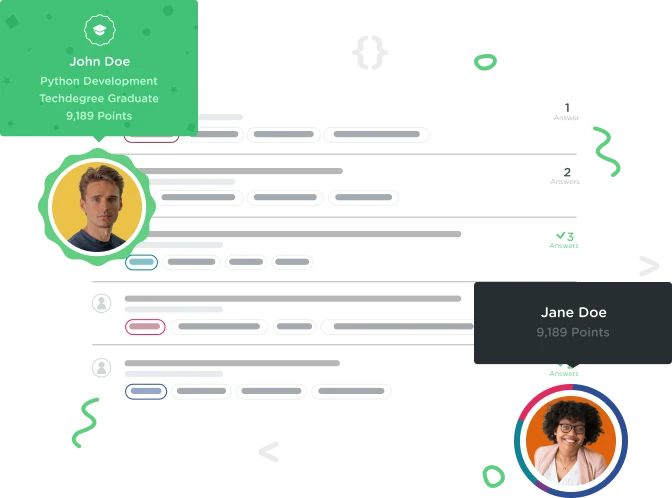

Silje Tellefsen
886 Pointscompare versions of random challenge
The first version is the right version to pass. Following a second version which will not pass. I do not understand why I cant use the second version? Can someone explain why this will be interpreted wrongly?
# right alternative
import random
start = 5
def even_odd(num):
return not num % 2
while start:
randint = random.randint(1, 99)
if even_odd(randint):
print("{} is even".format(randint))
else:
print("{} is odd".format(randint))
start -= 1
# questioned alternative
import random
start = 5
def even_odd(num):
return not num % 2
while start:
randint_1 = random.randint(1,99)
if randint_1 = even_odd(num):
print("{} is odd".format(randint_1))
else:
print("{} is odd".format(randint_1)
start -= 1
2 Answers

andren
28,558 PointsThere are quite a few issues in the second version. Most of which are found in this line of code:
if randint_1 = even_odd(num):
There are three issues with the line:
You use the = (assignment) operator instead of the == (equals) operator, which means that you aren't actually doing a comparison like you intend to do.
You make reference to a variable called
num
which does not exist inside yourwhile
loop.even_odd
does not return a number, it returns a Boolean value (True
orFalse
), so the comparison would always beFalse
.
The other issues with the code as a whole is that you print "{} is odd"
for both odd and even numbers, and you are missing a closing parenthesis on the second print
line.
If you have any further questions or want clarification on something I said then feel free to reply with more questions. I'll try to clarify anything I can.

Silje Tellefsen
886 PointsThanks. Regarding 2: If I put the def even_odd(num) inside the while loop, it could be used since it is defined here? (I thought that in python you did not need to define locally?)
Regarding 3, I still do not get why (given that num was defined inside the while loop) while start: randint_1 = random.randint(1,99) if randint_1 = even_odd(num): print("{} is odd".format(randint_1)) -will not take the boolean value as is done in while start: randint = random.randint(1, 99) if even_odd(randint): print("{} is odd".format(randint))