Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial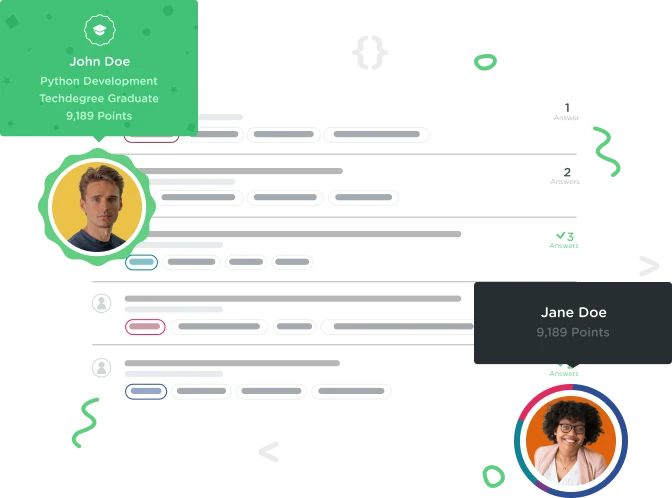
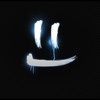
Grigorij Schleifer
10,365 PointscompareTo() question !
Hey folks,
I don´t understand this line of code:
if (equals(other)) {
return 0;
}
from the compareTo() method:
@Override
public int compareTo(Object obj) {
Treet other = (Treet) obj;
if (equals(other)) {
return 0;
}
int dateCmp = mCreationDate.compareTo(other.mCreationDate);
if(dateCmp == 0) {
return mDescription.compareTo(other.mDescription);
}
return dateCmp;
}
I understand that we cast "obj" to Treet, so the compiler knows that obj is a Treet and not mumbo jumbo. Then we use equals method from the Object class to compare "other" with something. My problem is that I can´t see this something in the code. I know that we compare "other" to a Treet but where do we define that Treet that compare with???
Something like this makes more sence to me:
Treet treet = new Treet();
if (treet.equals(other)) {
return 0;
}
Maybe someone can explain it to me ...
THANKS
Grigorij
1 Answer
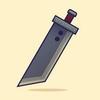
Allan Clark
10,810 PointsThis is a member method for the class Treet. Therefore whenever this method is called, it has to be called on a Treet Object and passed a parameter. The Treet it is being called on is what other will be compared to. Just putting equals implicitly implies that we are talking about the Treet it is being called on. It could also be explicitly written with the "this" keyword, like this:
if (this.equals(other)) {
return 0;
}
Lets say we have this code in our main method:
Treet firstTreet = new Treet();
Treet secondTreet = new Treet();
firstTreet.compareTo(secondTreet);
in this example we are comparing 'firstTreet' to 'secondTreet'. Because we are calling that method on a Treet Object, JVM implicitly knows that when we say equals() we are talking about the 'this' Object.
Hope this helps, let me know if I can clarify any further.
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsHi Allan,
it starts to make sence slowly.
Do I compare firstTreet to secondTreet here ?
Can you explain me the "this" keyword please??? I have dificulties to understand it.
I know that "this" is representing/referencing the current object from within an instance method or a constructor.
So if I use the compareTo() method and this method is defined in the class "A" and belongs to the object of that class. Will "this" keyword represent the object of class "A" ????
I have tried to make a litte programm but it gives me this runtime error:
Exception in thread "main" java.lang.ClassCastException: ClassB cannot be cast to ClassA
Here is the code:
ClassA:
Test class:
I hope my english is not so awfull like my code :)
Thanks a lot !!!
Grigorij
Allan Clark
10,810 PointsAllan Clark
10,810 PointsYes that seems correct. The 'this' keyword refers to the current instance of the class. So if you use the compareTo() method in ClassA, the this keyword will refer to whatever instance of the class you are currently working on, aka the one you called the method on. So lets say we had 2 variables of type ClassA lets call them var1 and var2. If i make the method call var1.compareTo(var2); all the code execution will happen inside the var1 instance, therefore the 'this' keyword will be referring to var1. Similarly, if we do the method call var2.compareTo(var1); the 'this' keyword will be referring to var2.
here is a good article with more information: http://www.javatpoint.com/this-keyword
The reason you are getting that error is casting requires inheritance, that is to say that one class must extend the other. To play around with this I would have ClassB extend ClassA, and also move your main method to a third class, ClassC maybe (just to get the start point out of the way of the models) .