Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial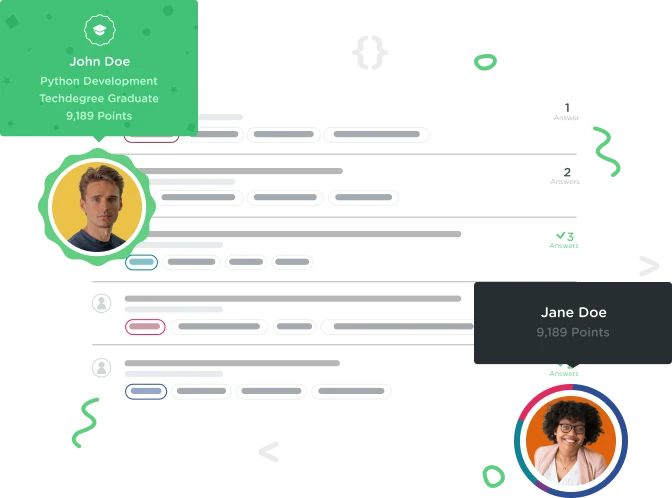

nick88p
5,716 PointsComparing arrays
Good day everyone,
I am trying to write a block of code that will compare two arrays and return a new array with any items not found in both of the original two arrays. This should work for numbers and words. For some reason I keep returning an empty array and I can't figure out why. Your help is much appreciated. Nick
function diff(arr1, arr2) {
var newArr = [];
var filteredOne = arr1.filter(firstFilter);
var filteredTwo = arr2.filter(secondFilter);
newArr = filteredOne.concat(filteredTwo);
return newArr;
}
function firstFilter(value,arr2) {
for (var i = 0; i<arr2.lenth; i++) {
return value !== arr2[i];
}
}
function secondFilter(value,arr1){
for (var i = 0; i<arr1.lenth; i++) {
return value !== arr1[i];
}
}
diff([1, 2, 3, 5], [1, 2, 3, 4, 5]);
2 Answers

LaVaughn Haynes
12,397 PointsFrom my understanding you can't use filter the way that you are using it. If you don't mind not using filter you can do it this way
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<script>
function getUniqueElements(a1, a2){
//var
var newArray = [];
//loop through the first array
for(var i=0; i < a1.length; i++){
//if the element not present in 2nd array
//push it to newArray
if( a2.indexOf(a1[i]) < 0 ){
newArray.push(a1[i]);
}
}
//return newArray
return newArray;
}
function diff(arr1, arr2){
var uniqueArray1 = getUniqueElements(arr1, arr2);
var uniqueArray2 = getUniqueElements(arr2, arr1);
return uniqueArray1.concat(uniqueArray2);
}
var theDifference = diff([1, 2, 3, 5], [1, 2, 3, 4, 5]);
console.log(theDifference);
</script>
</body>
</html>

nick88p
5,716 PointsThank you for your help. It is much appreciated. Nick.
Dan Oswalt
23,438 PointsDan Oswalt
23,438 PointsBah I was trying to find a way to do it with filter, this is better