Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial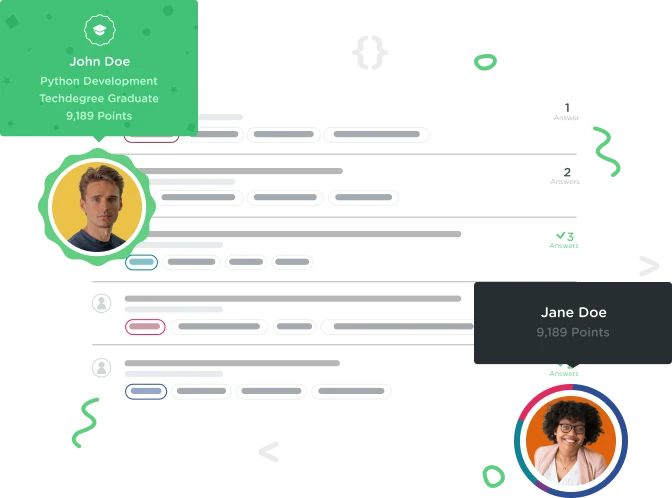
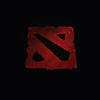
Wajeeh Hassan Qureshi
8,631 PointsComparitive function
I don't quite understand how the comparative function is used to sort out the number in the array. Also I don't understand this
return Math.random() - 0.5;
2 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsThe compare function gets called by the sort method for every pair of elements inside the array that is being sorted (most modern JavaScript environments will use shortcuts and optimizations here, but that's the basic idea).
The compare function returns a value. If the returned value is equal to 0, then the elements (a
and b
) are the same, and they're left in their places. If the returned value is less than 0, a
comes before b
in the sort. If the returned value is greater than 0, a
comes after b
in the sorted array.
You can read more about how the sort method works on MDN.
As for the second part of your question: Math.random() returns a random number between 0 and 1 (0 is inclusive, but 1 is not).
By subtracting 0.5
from the value returned by Math.random()
, you are essentially changing the range of the returned value.
For the smallest value that Math.random()
returns (0
) you will get -0.5
. And for the maximum value (0.9999...
) you will get (0.4999...
).
The code Math.random() - 0.5
will return such numbers, but you are using it inside the compare function of the sort method, so all that the code does is randomly (re)arrange the array. It will randomly return values that are less than, greater than or equal to 0, just as the sort method expects from the compare function, only they won't be making any sense as those values are randomly generated.

Dustin Morris
8,715 PointsIf different implementations take different shortcuts, are the results always the same? How can we anticipate and predict the results if we are unable to understand the mechanics of the function? I had the same question as John Adams. Any help would be great.

Dino Paškvan
Courses Plus Student 44,108 PointsThere are many different ways to sort an array, some of them work better for larger arrays, some of them work better for smaller ones. Different implementations might use a different sort based on the size of the array, or the type of data inside the array (Chrome used to use a version of QuickSort for numeric data, MergeSort for everything else, I don't know if that's still true).
Those things are out of our control when using JavaScript (unless you choose to write your own sorting function), but the end result will always be a sorted array.
You'll always get a sorted array (based on your rules in the compare function), no matter what sorting algorithm is used behind the scenes.
The only thing you really don't know is if the sort will be stable. I don't know if you're familiar with the term, but a stable sorting algorithm won't move around the elements with the same value.
Let's say you have an array like this: [5, 1, 6, 3, 1, 2]
. Sorted, it'll be: [1, 1, 2, 3, 5, 6]
.
A stable sort guarantees that the first element 1
in the sorted array was the first element 1
the algorithm encountered in the unsorted array, the second 1
is the second element 1
it encountered in the unsorted array and so on. An unstable sort doesn't guarantee that. It can happen, but not necessarily.
Since the JavaScript specification doesn't say if the sort should be stable, some implementations use unstable sorting, some use stable.
If this is a concern for you, you can turn any unstable sort into a stable sort. You need to store the original positions of elements, and then re-sort the same elements in the sorted array based on those positions. That way, you'll get exactly the same behaviour, regardless of the implementation of the sorting mechanism.
Wajeeh Hassan Qureshi
8,631 PointsWajeeh Hassan Qureshi
8,631 PointsThank you so much. This was very helpful.
John Adams
14,468 PointsJohn Adams
14,468 PointsI understand how the comparison is made, but I don't understand how (a, b) is related to the numbers in the array. For example if we have:
[1, 2, 3, 4, 5] and are using function (a, b)
how does JS pull the numbers and plug them into (a, b) ? Is there any order to this? Does it first compare 1 and 2, then 2 and 3? What about 1 and 3?
Hope that made sense.
Dino Paškvan
Courses Plus Student 44,108 PointsDino Paškvan
Courses Plus Student 44,108 PointsIt really depends on the implementation. How the compare function is called is browser/platform specific — it's not a part of the ECMAScript (JavaScript) standard.
The most basic way would be for the engine to call that function on every possible combination of array element pairs, but that's very slow. In reality, different implementations take different shortcuts.