Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial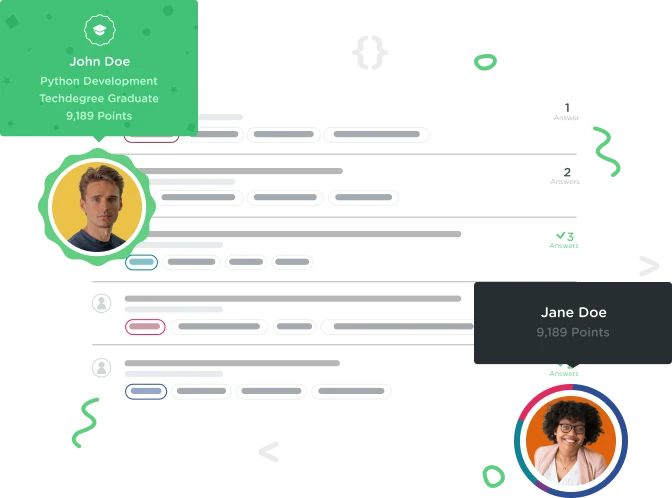
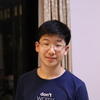
Thanitsak Leuangsupornpong
7,490 PointsCompilation Fail in DecreaseHealth Method.
In the video there is no return keyword in the DecreaseHealth method, but when I complie I got this messages. I solved by typed return inside DecreaseHealth method, and also how to get rid of this warning. Much Appreciate!
Game.cs(17,10): warning CS0219: The variable `path' is assigned but its value is never used
Invader.cs(65,14): error CS0161: `TreehouseDefense.Invader.DecreaseHealth(int)': not all code paths return a value
Compilation failed: 1 error(s), 1 warnings
2 Answers
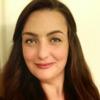
Jennifer Nordell
Treehouse TeacherHi there! First, this would be much easier to help you with if we could see your code. You could either post it here using the instructions found in the Markdown Cheatsheet link at the bottom of the "Add an Answer" section or you could link us a snapshot to your workspace. You can create a snapshot by clicking the camera icon on the upper right hand side of your workspace.
My best guess as to why you've received the error and fixed it with a return
statement is because the DecreaseHealth
function was not assigned a return type of void
. If the return type of the method is not void
the compiler will expect the return of a value on all possible logical paths.
As for the warning, I'm actually going to need to see your code. But somewhere you've declared a variable named path
, but then never used it.
Hope this explains the return error, but I look forward to seeing your code so that I can help with the warning!
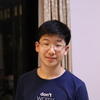
Thanitsak Leuangsupornpong
7,490 PointsYou right! I should type void like the video but I typed int instead. Thank you!!!
Thanitsak Leuangsupornpong
7,490 PointsThanitsak Leuangsupornpong
7,490 PointsThank You very much!
Here my code. On Game class
On Invader class
Much Appreciate