Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial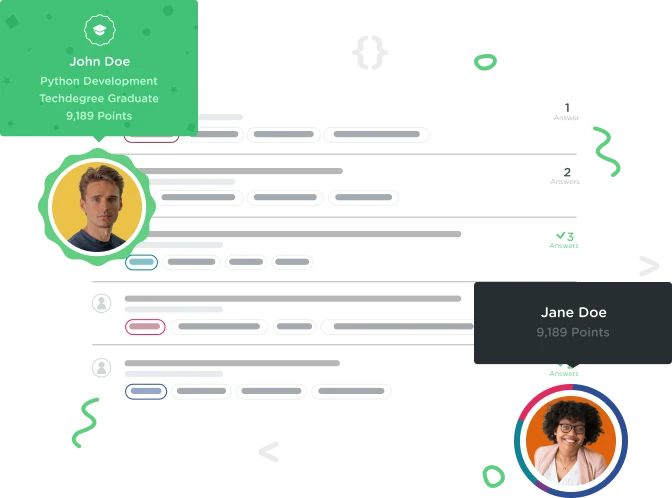

hankamamut
3,177 Pointscompile error
First I tried version 1 because I think we need to pass two paramaters to the constructor: Number of sides which is hardcoded to 4 and the side length. This didn't work, so I tried the version that was recommended in the previous post and got an error the same error as for Version 1.
Can you please explain how the patameters need to be listed in the subclass constructor? The lectures shows that two parameters are passed from the parent class to the child constructor because the parent class constructor uses two parameters.
Thanks.
Version 1:
namespace Treehouse.CodeChallenges { class Polygon { public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square ( int numSides, int sideLenght) : base (4,sideLenght)
{
SideLength = sideLenght;
}
}
}
Version 2:
namespace Treehouse.CodeChallenges { class Polygon { public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square ( int sideLenght) : base (4)
{
SideLength = sideLenght;
}
}
}
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square ( int sideLenght) : base (4)
{
SideLength = sideLenght;
}
}
}
3 Answers
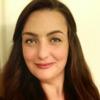
Jennifer Nordell
Treehouse TeacherHi hankamamut ! You're actually doing really well here! In fact, your last version of the code would pass except for one thing: you continually misspell the word "length" as "lenght". Notice the reversal of the "t" and "h". So, if I correct the spelling in the Square class, it passes! Take a look:
class Square : Polygon
{
public readonly int SideLength;
public Square ( int sideLength) : base (4)
{
SideLength = sideLength;
}
}
Double-check your spelling as that's why you're getting errors. Happy coding!

hankamamut
3,177 PointsThank you very much. That helped and the code compiled. However, I still don't understand why the Square constructor needs only one parameter. In my mind it should have two parameters because it inherits numSides parameter from the Polygon class and adds its own parameter sideLength. Why is my line of thinking wrong? Thanks in advance.
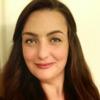
Jennifer Nordell
Treehouse TeacherIt's because a polygon can only have one number to represent the sides. The shape either has 3 sides or 5. It's either going to be a triangle or a pentagon. But not all polygons are regularly shaped and can have different side lengths. A rectangle for example could have sides of 2 inches and top and bottom of 3 inches. However, a square by definition has equal side lengths. This is why we include the base: 4 to give a default of 4 sides when creating a square as a square has 4 sides by definition. When we create our new polygon all we need to tell it is how many sides the polygon has so that we know what kind of shape we're dealing with.
So when creating a square it sends in the 4 sides to the Polygon, and we send in the side length to the Square.
Hope this helps!

hankamamut
3,177 PointsYou are saying "So when creating a square it sends in the 4 sides to the Polygon, and we send in the side length to the Square" and that's why I'd expact the construct to be public Square ( int numSide, int sideLength) : base (4) instead of public Square ( int sideLength) : base (4)
Similar to MapLocation (int x, int y, Map map) : base (x,y)