Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial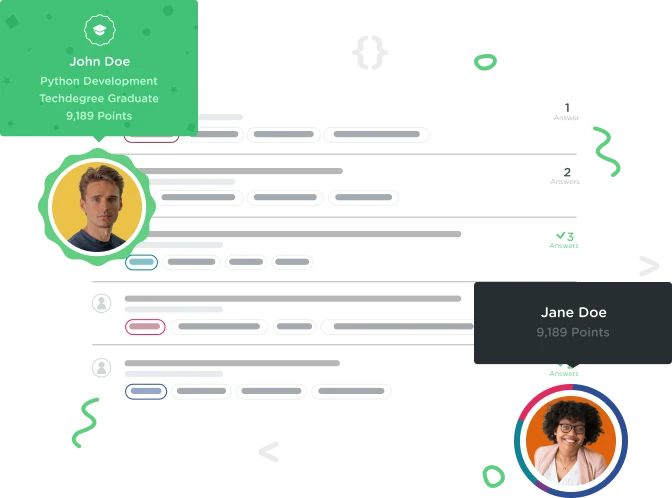
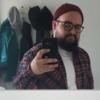
Gabe Olesen
1,606 PointsCompile Error?
Hey All,
Am I being stupid?
package com.teamtreehouse;
import com.teamtreehouse.model.SongBook;
import com.teamtreehouse.model.Song;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.HashMap;
import java.util.Map;
public class KaraokeMachine {
private songBook mSongBook;
private BufferedReader mReader;
private Map<String, String> mMenu;
public KaraokeMachine(SongBook songBook) {
mSongBook = songBook;
mReader = BufferedReader(new InputStreamReader(System.in));
mMenu = new HashMap<String,String>();
mMenu.put("add", "Add a new song to the song book");
mMenu.put("quit", "Give up. Exit the program");
}
private String promptAction() throws IOException {
System.out.printf("Thre are %d songs available. Your options are: %n",
mSongBook.getSongCount());
for (Map.Entry<String, String> option : mMenu.entrySet()) {
System.out.printf("%s - %s %n",
option.getKey(),
option.getValue());
}
System.out.print("What do you want to do: ");
String choice = mReader.System.readLine();
return choice.trim().toLowerCase();
}
public void run() {
String choice = "";
do {
try {
choice = promptAction();
switch(choice) {
case "add":
Song song = promptNewSong();
mSongBook.addSong(song);
System.out.printf("%s added! %n%n", song);
break;
case "quit":
System.out.println("Thanks for playing");
break;
default:
System.out.printf("Unknown choice: '%s'. Try again. %n%n%n",
choice);
}
} catch(IOException ioe) {
System.out.println("Problem with input");
ioe.printStackTrace();
}
} while(!choice.equals("quit"));
}
private Song promptForNewSong() throws IOException{
System.out.print("Enter the artist's name: ");
String artist = mReader.readLine();
System.out.print("Enter the title: ");
String title = mReader.readLine();
System.out.print("Enter the video URL: ");
String videoUrl = mReader.readLine();
return new Song(artist, title, videoUrl);
}
}
Error:
Picked up JAVA_TOOL_OPTIONS: -Xmx128m
Picked up _JAVA_OPTIONS: -Xmx128m
./com/teamtreehouse/KaraokeMachine.java:13: error: cannot find symbol
private songBook mSongBook;
^
symbol: class songBook
location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:20: error: cannot find symbol
mReader = BufferedReader(new InputStreamReader(System.in));
^
symbol: method BufferedReader(InputStreamReader)
location: class KaraokeMachine
./com/teamtreehouse/KaraokeMachine.java:35: error: cannot find symbol
String choice = mReader.System.readLine();
^
symbol: variable System
location: variable mReader of type BufferedReader
./com/teamtreehouse/KaraokeMachine.java:46: error: cannot find symbol
Song song = promptNewSong();
^
symbol: method promptNewSong()
location: class KaraokeMachine
4 errors
2 Answers

Felix Sonnenholzer
14,654 PointsHi,
there are a few minor mistakes, that's why it won't compile.
First of all, you need the new
keyword to instantiate an Object. You are missing the new
in the constructor.
mReader = new BufferedReader(new InputStreamReader(System.in));
Second, in your promptAction()
method you are not using the reader correctly.
You need to remove the System
String choice = mReader.readLine();
Third, in your run()
method you are trying to call a promptNewSong()
method. The method is called promptForNewSong()
Song song = promptForNewSong();
I hope that's all, if there's more I didn't see it :)
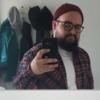
Gabe Olesen
1,606 PointsThanks Felix,
It was compiling fine earlier this afternoon, I did drag the .java file by mistake into the root this may of caused the issues