Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial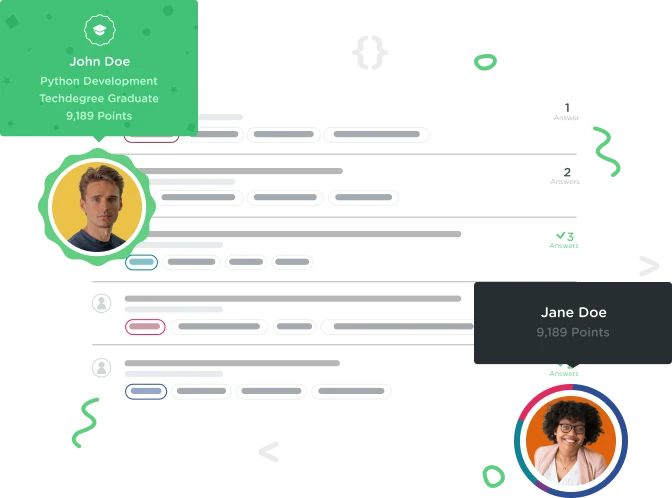

Herman Vicens
12,540 PointsCompile error !
Hey, Me again!
I am getting compile errors and cannot find the reason. The error is as if I'm missing an import statement but I'm not. The Map initialization statement is giving me an error and sintactically, I can't find a problem with it. Looked into the documentation in Oracle, and verified that there were no syntax errors. It is a new topic for me and it is dificult to get rid of these errors. Any suggestions?
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
import java.util.HashMap;
import java.util.Map;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> mapCount = HashMap<String, Integer>();
for (BlogPost catPost : mPosts) {
for (String catCount : catPost.getCategory()) {
Integer count = mapCount.get(catCount);
if (count == null) {
count = 0;
}
count ++;
mapCount.put(catCount, count);
}
}
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
}
2 Answers
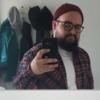
Gabe Olesen
1,606 PointsHey Herman Vicens,
I found your error!
Your method getCategory counts, you don't need two for-loops because the second for-loop already returns a string so there's no need to create a String for-loop. All we want to do is loop over all of the created BlogPosts and return what category they are and their count.
So, as getCategory() method already returns a string on this line for (BlogPost catPost : mPosts) we're looping through all of the created BlogPosts. Next we to assign count to get the category so we do Integer count = mapCount.get(catPost.getCategory());.
Great,
Whenever you store a new key and value into a map it will return null (you can test this in Jshell on workspaces). So we will create a if statement to check if the category it's there or if it's being added FOR the first time it will return null so we will set the count to 0. Then we will increment the count to see Hey! there's a Tech category lets add 1.
Once we've incremented, we will put what we've gathered from the If loop into the map hence the put() method.
For demonstration purposes you can put a printf statement to see if it works and click preview on the challenge to see if it works. You will see the output below;
That's pretty much it!
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> mapCount = new HashMap<String, Integer>();
for (BlogPost catPost : mPosts) {
Integer count = mapCount.get(catPost.getCategory());
if (count == null) {
count = 0;
}
count ++;
mapCount.put(catPost.getCategory(), count);
}
System.out.printf("Catergories: %s", mapCount);
return mapCount;
}
Output:
Catergories: {Tech=3, Entertainment=1, Health=3, Science=1, Food=2}
Happy coding!
~Gabe

Herman Vicens
12,540 PointsThanks Gabe! It worked. The error I was getting no was even close to what you found. Thanks again for your support!!
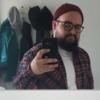
Gabe Olesen
1,606 PointsNo problem !
Gabe Olesen
1,606 PointsGabe Olesen
1,606 PointsHey Herman,
What's the compile error you're getting?!
~Gabe