Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial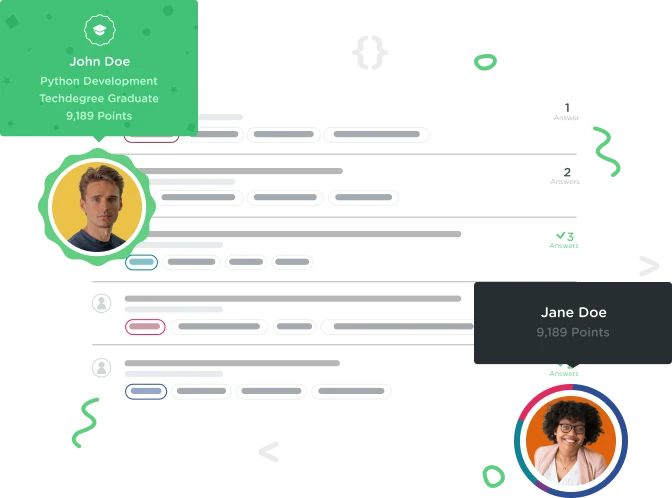

Ryan West
5,639 PointsCompile problem with no error
in swift i'm getting a compile error but I've ran it in the playground and everything works properly, what am I forgetting on this step?
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
init(title: String, author: String) {
self.title = title
self.author = author
self.tag = Tag(name: "swift")
}
func description() -> String {
return "\(title) by \(author). Filed under \(tag)"
}
}
let firstPost = Post(title: "iOS Development", author: "Apple")
let postDescription = firstPost.description()
2 Answers

Joe Beltramo
Courses Plus Student 22,191 PointsThere are two things incorrect here.
First: Even though your first task passed, the actual intention was for your init
method to accept a tag value as a Tag: i.e.
...
init(title: String, author: String, tag: Tag) { ... }
Next when you reference the tag in the description output, it is looking for the name of the tag so try:
return "... Filed under \(tag.name)"
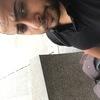
Ahmed Elkasrawi
2,756 PointsGuys, do you see anything wrong with the code below? it works find on Xcode but the exercise won't compile it.
Thanks
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
init(title: String, author: String, tag: Tag) {
self.title = title
self.author = author
self.tag = tag
}
func description() -> String {
return "\(title) by \(author). Filled under \(tag.name)"
}
}
let firstPost = Post(title: "iOS Development", author: "Apple", tag: Tag(name: "swift"))
let postDescription = firstPost.description()

Ryan West
5,639 PointsHey Ahmed,
Looks like you spelt "Filled" wrong, should be "Filed". Just checked it and it works properly. Try that and let me know what you get.
Cheers, Ryan
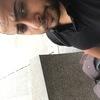
Ahmed Elkasrawi
2,756 PointsThanks Ryan, that was it.. I was only looking for syntax errors and overlooked my own typos..
Ryan West
5,639 PointsRyan West
5,639 Pointshow does the body look in the init method
currently I have:
init(title: String, author: String, tag: Tag){ self.title = title self.author = author //Part i'm stuck: self.tag = Tag(name: "Empty string")??
Joe Beltramo
Courses Plus Student 22,191 PointsJoe Beltramo
Courses Plus Student 22,191 PointsSince you are expecting tag to already be set as a Tag in instantiation, you can easily & safely just do
self.tag = tag
But remember to also update your
firstPost
variableRyan West
5,639 PointsRyan West
5,639 PointsThanks a bunch Joe, that make more sense, I don't know why I was over complicating it.
Joe Beltramo
Courses Plus Student 22,191 PointsJoe Beltramo
Courses Plus Student 22,191 PointsAlways better to ask than to bang your head on the desk :]