Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial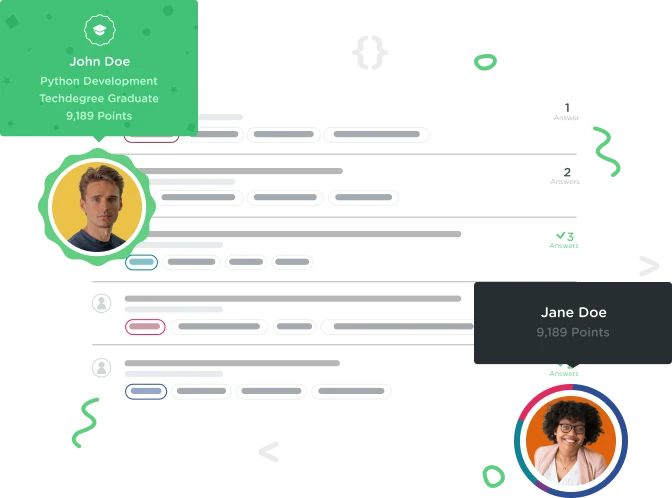
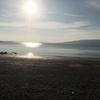
Arran C
880 PointsCompiler error
I'm getting this error and have no idea what i've done wrong:
error:
"Program.cs(59,21): error CS0136: A local variable named minutes' cannot be declared
in this scope because it would give a different meaning to
minutes', which is already used in a `child' scope to denote something else "
code :
using System;
namespace Treehouse.FitnessFrog
{
class Program
{
static void Main()
{
int runningTotal = 0;
bool keepGoing = true;
while (keepGoing)
{
// Prompt the user for minutes exercised
Console.Write("Enter how many minutes you have exercised or type \"quit\" to exit: ");
string entry = Console.ReadLine();
if(entry == "quit")
{
keepGoing = false;
}
else
{
try
{
int minutes = int.Parse(entry);
if(minutes <= 0)
{
Console.Write(minutes + " is not an acceptable value. ");
continue;
}
else if(minutes <= 10)
{
Console.Write("Better than nothing I suppose. ");
}
else if(minutes <= 30)
{
Console.Write("Hell yeah! ");
}
else if(minutes <= 60)
{
Console.Write("Am I seeing a warrior in training? ");
}
else
{
Console.Write("Stop showing off! But don't stop exercising though, it's good for you! ");
}
}
catch(FormatException)
{
Console.Write("That is not a valid input. ");
continue;
}
int minutes = int.Parse(entry);
runningTotal = runningTotal + minutes;
// Add minutes exercised to total
// Display total minutes exercised to the screen#
Console.WriteLine("You've entered " + runningTotal + " minutes");
}
// Repeat until user quits
}
Console.WriteLine("Goodbye");
}
}
}
1 Answer
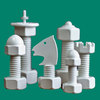
Steven Parker
231,269 PointsTry declaring "minutes" before the "try", and then inside the try simply assign it without declaring it again.
Also remove the second declaration and initialization that is currently after the "catch".
Arran C
880 PointsArran C
880 PointsI tried doing that but no luck
EDIT
I messed around with it and eventually after putting
runningTotal = runningTotal + minutes;
in the try block, it worked :)
Steven Parker
231,269 PointsSteven Parker
231,269 PointsThat particular line should be fine wherever you place it. The "try" block mainly needs to contain the "int.Parse" line.