Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial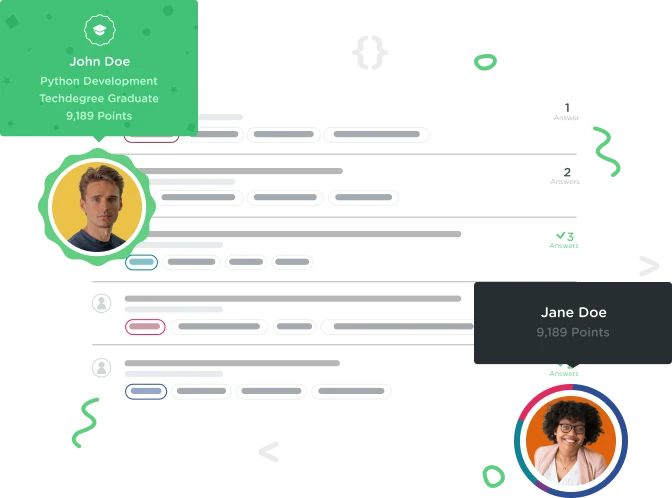

Tojo Alex
Courses Plus Student 13,331 PointsCompiler error
I'm not certain exactly were I went wrong:
Frog.cs(7,30): error CS0102: The type Treehouse.CodeChallenges.Frog' already contains a definition for
_numFliesEaten'
Frog.cs(5,21): (Location of the symbol related to previous error)
Frog.cs(12,10): error CS1525: Unexpected symbol }', expecting
;'
Compilation failed: 2 error(s), 0 warnings
namespace Treehouse.CodeChallenges
{
public class Frog
{
private int _numFliesEaten;
public NumFliesEaten _numFliesEaten
{
get
{
return _numFliesEaten
}
set
{
_numFliesEaten = value;
}
}
}
}

Chris Kopcow
2,852 PointsTo solve Frog.cs(7,30): error CS0102: The type Treehouse.CodeChallenges.Frog' already contains a definition for_numFliesEaten'
, you have to rename the property.
You already initialized _numFliesEaten
as an int
field. You can't also name a property _numFliesEaten
too because there's already something that's called that.
So
namespace Treehouse.CodeChallenges
{
public class Frog
{
private int _numFliesEaten;
public int NumFliesEaten
{
get
{
return _numFliesEaten;
}
set
{
_numFliesEaten = value;
}
}
}
}
For the other error, you just forgot to add a semicolon to the end of your getter.
Hope this helps!
Shadab Khan
5,470 PointsShadab Khan
5,470 PointsHi Tojo,
The syntax of a public property in C# is : public [type of property] [Property name] In your case is should be : public int NumFliesEaten.
So your code should look like so
If not for challenge, you could also use auto-implemented properties in C# like so. It's very clean and concise :)
All the best and happy coding :)