Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial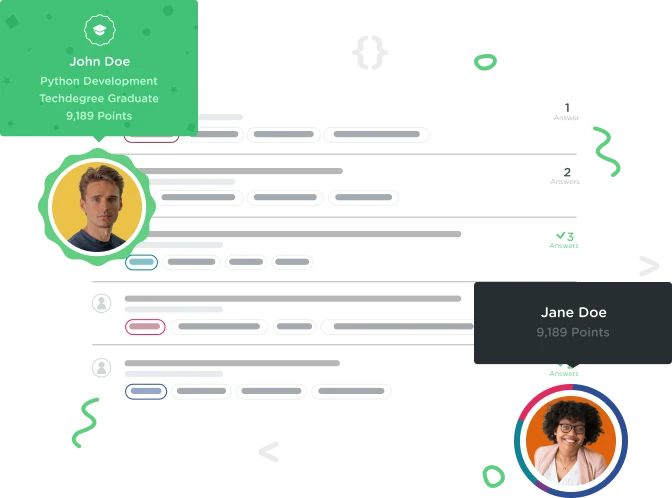

elomaur
5,276 PointsCompiler Error
Here is my code for path.cs
namespace TreehouseDefense
{
class Path
{
private readonly MapLocation[] _path;
// using underscore is a common convention in C#. It is helpful for distinguishing between instance variables anad metho variables. If we didn't have the underscore, noth path variables will have to be named differently or we would use this.path = path instead of _path = path
public Path(MapLocation[] path)
{
_path = path;
}
public MapLocation GetLocationAt(int pathStep)
{
return (pathStep < _path.Length) ? _path[pathStep] : null;
}
}
}
and here is the code for game.cs
using System;
namespace TreehouseDefense
{
class Game
{
public static void Main()
{
Map map = new Map(8, 5);
try
{
MapLocation[] path = {
new MapLocation(0, 2, map),
new MapLocation(1, 2, map),
new MapLocation(2, 2, map),
new MapLocation(3, 2, map),
new MapLocation(4, 2, map),
new MapLocation(5, 2, map),
new MapLocation(6, 2, map),
new MapLocation(7, 2, map),
};
}
catch(OutOfBoundsException ex)
{
Console.WriteLine(ex.Message);
}
catch(TreehouseDefenseException ex)
{
Console.WriteLine("Unhandled TreehouseDefenseException");
}
catch(Exception)
{
Console.WriteLine("Unhandled Exception");
}
}
}
}
I am getting the following warnings when I compile the code
Game.cs(13,27): warning CS0219: The variable `path' is assigned but its value is never use
d
Game.cs(31,44): warning CS0168: The variable `ex' is declared but never used
Tower.cs(7,13): warning CS0219: The variable `tower' is assigned but its value is never us
ed
Compilation succeeded - 3 warning(s)
Please explain the reason for the warning as my code is the same as the code shown in the video.
2 Answers

Samuel Savaria
4,809 PointsThese aren't errors, these are warnings. Your code will compile and run without any problem, the console is simply trying to warn you about possible problems.
warning CS0219: The variable `path' is assigned but its value is never used
Here you created a new path but never used it anywhere in your code. The code will still work and you will use that variable in the course but right now the console doesn't understand why you created a variable that you don't use and is warning you that you might have forgotten to reference it somewhere.
Game.cs(31,44): warning CS0168: The variable `ex' is declared but never used
Same thing here. You created a variable that you aren't using yet. Like before, you will use it later on in this course.

elomaur
5,276 PointsThank you for the response. I was curious as to why I was receiving these warnings when the code was the same as the code used in the video. I apologize for the misleading title. I am indeed aware that these are not errors.