Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial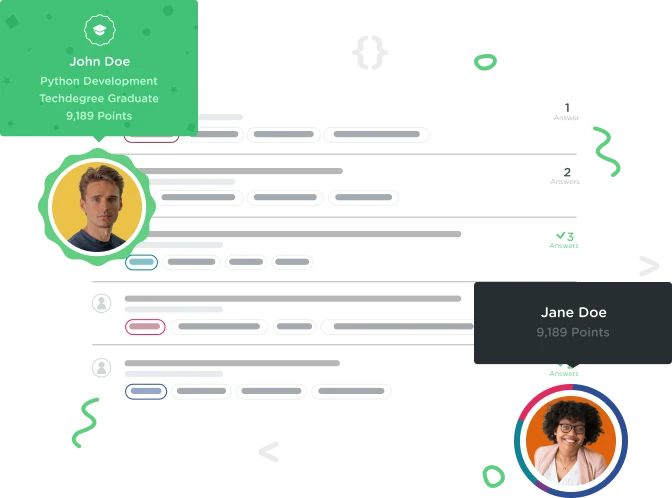
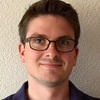
Anthony Lafont
17,075 Pointscompiler error: 'new()' is unavailable in Swift: use object initializers instead, even in the teachers note files
There is an error in the class HorseRace at the line raceTracker.startTime = NSDate.new() It says "'new()' is unavailable in Swift: use object initializers instead" Do you know how to fix it?
import Foundation
// Participants
struct Horse {
func giddyUp() {}
}
struct Car {
func vroomVroom() {}
}
struct RaceCar {
func readySetGo() {}
}
// Tracker
class Tracker {
var laps: Int = 0
var startTime: NSDate?
var lapFirst: Horse?
var winner: Horse?
}
// Races
class Race {
var laps: Int = 0
let raceTracker: Tracker = Tracker()
func start() {
// Some set up
}
func updateProgress() {
}
func end() {
// Some tear down
}
}
class HorseRace: Race {
let participants: [Horse]
init(laps: Int, horses: [Horse]) {
self.participants = horses
super.init()
self.laps = laps
}
override func start() {
print("Starting Race!")
raceTracker.startTime = NSDate.new()
for horse in participants {
horse.giddyUp()
}
}
override func updateProgress() {
raceTracker.laps += 1
raceTracker.lapFirst = participants.first
print("Progress updated!")
}
override func end() {
print("And the winner is...\(participants.first)")
raceTracker.winner = participants.first
}
}
// Usage
let horse1 = Horse()
let horse2 = Horse()
let horse3 = Horse()
let race = HorseRace(laps: 4, horses: [horse1, horse2, horse3])
race.start()
race.updateProgress()
race.end()
1 Answer
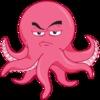
Kieran Black
9,139 PointsHi Anthony,
Instead of
raceTracker.startTime = NSDate.new()
Try
raceTracker.startTime = NSDate()
Hope that helps
KB