Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial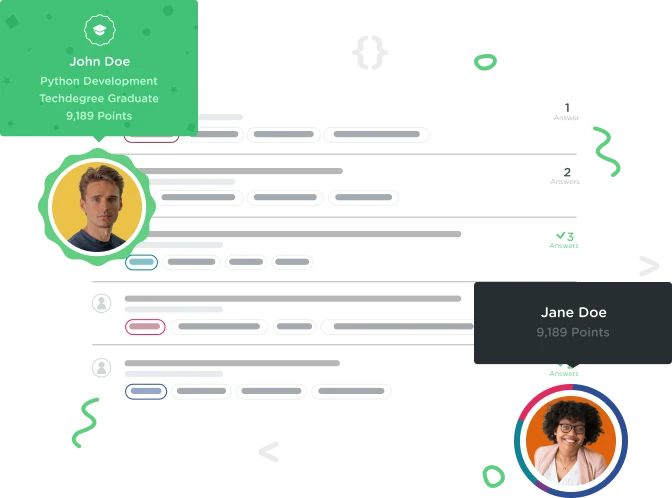

Matthew Egan
1,437 Pointscompiler error regarding return types
I am currently working on the final coding project in the first half of the C# Objects course, and it is asking me to make a parameter to assign an input integer to a readonly value. I used an identical method to the code found not 10 lines above it, but I still found an error in my code. Any suggestions?
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public readonly int ReactionTime;
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
public bool EatFly(int distanceToFly)
{
return TongueLength >= distanceToFly;
}
public reactionTime(int reactTime)
{
ReactionTime = reactTime;
}
}
}
2 Answers
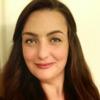
Jennifer Nordell
Treehouse TeacherHi there, Matthew! You did just fine on adding the ReactionTime property! Step 2 is asking for you to add a new parameter to the constructor for the class Frog
. But what you'e done is add a new method. The reason you're getting the compiler error regarding the return is because every method aside from the constructor needs a return type. But the method you added doesn't have a return type and will cause it to not compile. But again, the method is not needed and can be safely deleted.
Currently the constructor accepts one integer named tongueLength
. We then use that to set the value of our read only property to what was sent in. The only thing it's asking you to do is add a second parameter to the constructor that takes another number. This one will be used in the exact same way as the TongueLength = tongueLength;
line is used.
I feel like you can get it with these hints, but let me know if you're still stuck!

Matthew Egan
1,437 PointsThanks Jennifer! I feel kinda silly for not realizing that sooner. I guess I need to review this stuff a little harder so that I can really get it. I saw the constructor and thought it was its own method, and thus made a separate method that had the same value in it. Again, it was a dilly mistake. I figured it out, and I am now done with the methods, but I think I'm going to brush up on the content again to be safe.