Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial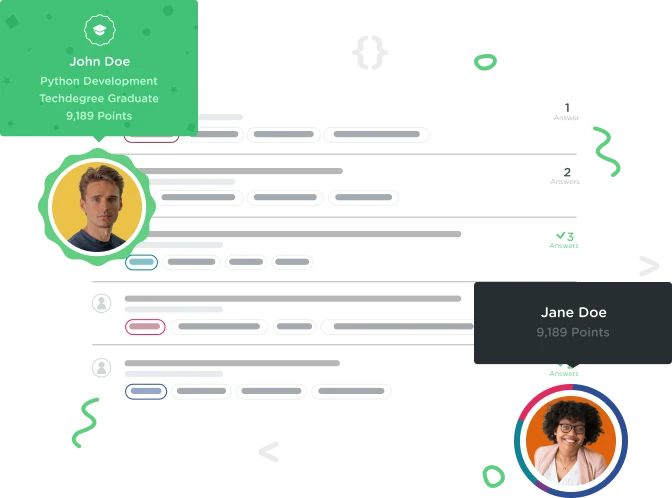

Aryaman Dhingra
3,536 PointsCompiler not showing errors?!
It's saying there is an error, and to check the compiler to see it but it's not being shown. I tried in Xcode and I'm not getting any error there.
struct Tag {
let name: String
}
struct Post {
var title: String
var author: String
var tag: Tag
init(name: String, author: String, title: String) {
self.tag = Tag(name: name)
self.author = author
self.title = title
}
func description() -> String {
return "\(title) by \(author) . Filed under \(tag)"
}
}
let firstPost = Post(name: "Hi", author: "Bye", title: "Lie")
let postDescription = firstPost.description
1 Answer

Greg Kaleka
39,021 PointsHi Aryaman,
That error message is not helpful! There are a few things you're doing wrong in your code, and a few things that you should be doing differently (but aren't wrong per se):
- There's no need to implement a custom init method. Swift will create an init method on its own. If you don't use the custom init you created, you'll need to pass a Tag object, rather than just the tag name, but still - I think it's clearer than passing a name that isn't actually a Post's name. In a real blog application, you would probably already have tags created, so you don't want to have to create new ones any time you create a post. You'll also have to re-order the arguments to match how you declare them in the struct (title, author, tag).
- In your description method, you need to use tag.name, otherwise you'll get the whole Tag object (it will look like this: "...filed under Tag(name: "Hi")". That's not what you want)
- You have an extra space in your description (before the period). The code challenge is picky about that.
- When you call the
description
method, you need parentheses.firstPost.description()
, notfirstPost.description
Seems like a lot of stuff, but I'd say you were actually really close! Hope this all makes sense. Let me know if you have questions or don't quite understand how something is working.
Cheers
-Greg
struct Tag {
let name: String
}
struct Post {
var title: String
var author: String
var tag: Tag
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title: "Lie", author:"Apple", tag: Tag(name: "hi"))
let postDescription = firstPost.description()