Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial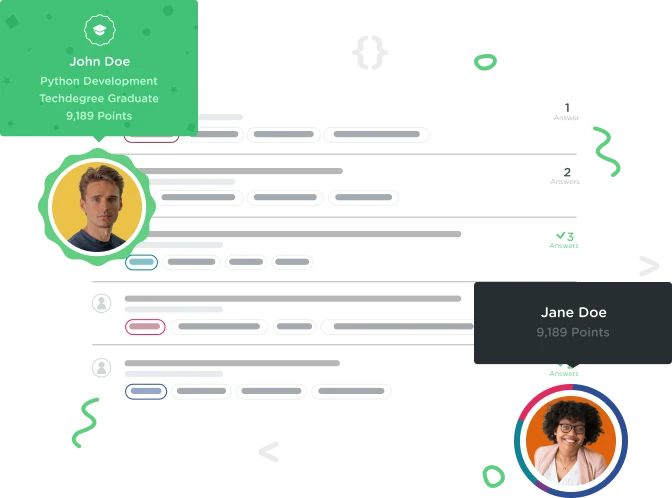

Habibur Rahman Dipto
8,496 PointsCompleted the extra question credit need to review.
Question: Try to build the opposite of this game. Make a game where the computer tries to guess your secret number. You'll need to come up with a way to tell the computer if it's too high, too low, or if it guesses the number!
Here is the answer.
import random
guess_num = random.randint(1, 10)
guessed_num = []
print("""Wellcome to the guessing number game. Guess a number.
type 'high' the computer guess is high.
type 'low' if the computer guess is low
type 'yes' if the computer guess is correct""")
while len(guessed_num) < 5:
print("My number is {}".format(guess_num))
secret_num = input("Am i right? ")
if guess_num not in guessed_num:
if secret_num == 'high':
guess_num = random.randint(guess_num + 1, 10)
continue
elif secret_num == 'low':
guess_num = random.randint(1, guess_num - 1)
continue
elif secret_num == 'yes':
print("Yes I win!!!")
break
guessed_num.append(guess_num)
1 Answer

Ira Bradley
12,976 PointsA couple of things -> it would be nice if you mention the range of numbers that a player can guess. It would be nice to allow the human to think of a number before the computer begins guessing. If you want your program to efficiently guess the number it should start with 5 every time. Currently when the computer guess too high the human must type "low" and vice versa which seems backwards. It would be good to add try and except blocks to the program incase of wrong input on the part of the user. Currently if the user enters wrong input the program doesn't break but it stops working correctly.