Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial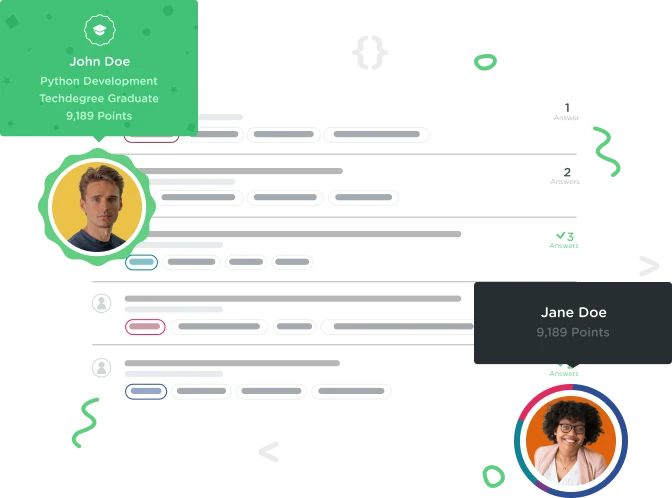
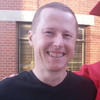
Thad Roe
10,687 PointsCompleted the Multidimensonal array challenge, but still don't think I did it right
The last part of this (echoing in the line items) really gave me trouble. I finally got the correct output, but don't think I did it in the most concise way or as intended.
Was there a better way to do this? I couldn't seem to concatenate both the name and email values on one line.
Here's what I ended up doing:
<?php
//edit this array
$contacts[] = [
'name' => 'Alena Holligan',
'email' => 'alena.holligan@teamtreehouse.com',
];
$contacts[] = [
'name' => 'Dave McFarland',
'email' => 'dave.mcfarland@teamtreehouse.com',
];
$contacts[] = [
'name' => 'Treasure Porth',
'email' => 'treasure.porth@teamtreehouse.com',
];
$contacts[] = [
'name' => 'Andrew Chalkley',
'email' => 'andrew.chalkley@teamtreehouse.com',
];
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>" . $contacts[0]['name'];
echo " : " . $contacts[0]['email'] . "</li>\n";
echo "<li>" . $contacts[1]['name'];
echo " : " . $contacts[1]['email'] . "</li>\n";
echo "<li>" . $contacts[2]['name'];
echo " : " . $contacts[2]['email'] . "</li>\n";
echo "<li>" . $contacts[3]['name'];
echo " : " . $contacts[3]['email'] . "</li>\n";
echo "</ul>\n";
1 Answer
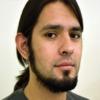
Carlos Alberto Del Cueto Carrejo
13,817 PointsThere is nothing wrong on the way that you resolved this task. In the end it is just what you needed. Another way to complete the challenge I would have used a foreach loop to print the results. As in :
<?php
echo "<ul><br>";
foreach($contacts as $contact){
echo "<li>" . $contact['name'] . " : " . $contact['email'] . "</li><br>";
}
echo "<ul>";
?>
Thad Roe
10,687 PointsThad Roe
10,687 PointsThanks for the reply! I'm not quite at the foreach stage yet, but nice to have a peek at how that makes it more concise.
After some sleep and looking at your example vs. mine, I realize I was originally making a concatenation error.
I realize now to keep each li echo in one statement I should have done this: