Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial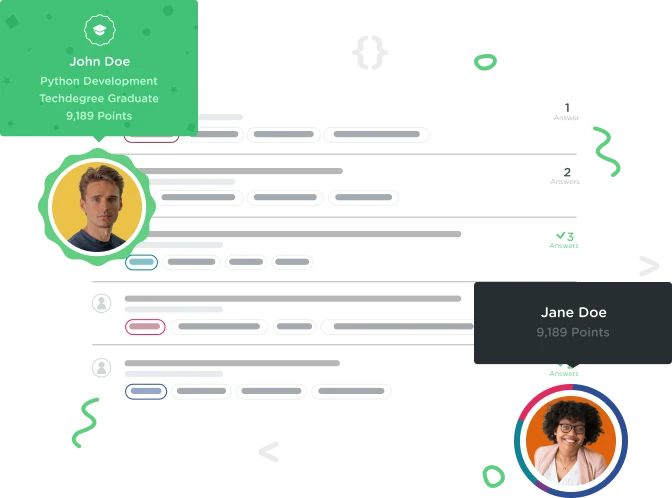

Nas Jones
7,849 PointsCompletely confused
I watched the setter video before this and i was lost. set owner(owner) {
this._owner = owner;
console.log(setter called: ${owner}
);
}
If you look at this code i see four different places where "owner" was used before it was logged in the console, why were they used in each instance?. I also don't understand why the "_" was necessary. Also, in this challenge i'm confused as well, all i got out of it was an if statement i don't know what to do further. Also, if this can be explained simply that would help me better instead of using complex words and code
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
set major(major) {
this._major = major;
if (level == 'Senior' || level == 'Junior') {
}
}
}
var student = new Student(3.9, 60);
1 Answer
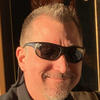
Peter Vann
36,425 PointsHi Nas!
Sorry for the late response. Just saw this.
As to your first point:
This:
set owner(owner) {
this._owner = owner;
console.log(`setter called: ${owner}`);
}
Could just as easily be coded:
set owner(tmpParam) {
this._owner = tmpParam;
console.log(`setter called: ${tmpParam}`);
}
or even:
set owner(x) {
this._owner = x;
console.log(`setter called: ${x}`);
}
The parameter name is arbitrary, but "owner" makes sense in this context (albeit potentially confusing).
The preceding "_" denotes a "private" object property or method.
More info:
https://www.thoughtco.com/and-in-javascript-2037515
Obviously, this would pass task 1:
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
set major(major) { // Task 1, which you already got
}
}
var student = new Student(3.9, 60);
For this: Inside the major() setter method, set the student's major to a backing property "_major".
You would do this:
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
set major(major) {
this._major = major; // First part of Task 2, which you already got
}
}
var student = new Student(3.9, 60);
For this: If the student's level is Junior or Senior, the value of the backing property should be equal to the parameter passed to the setter method. If the student is only a Freshman or Sophomore, set the "_major" backing property equal to 'None'. Let me see if I can rephrase it more simply.
If the getter "level" (this.level) returns (is equal to) Freshman or Sophomore, change this._major to "None" otherwise leave it as the major parameter (don't change it).
I can show you that code if you can't solve it from the description now.
Please let me know.
I hope that helps.
Stay safe and happy coding!
Nas Jones
7,849 PointsNas Jones
7,849 PointsHello thanking you for responding and trying to help i truly appreciate it. I passed the challenge thanks to your help i understood what you were saying for the most part. But i still kind of don't get it, i remember how the instructor in previous videos said how this can be confusing and i am confused. What is the goal of getters and setters in this case? what are we trying to achieve?. set owner(x) { this._owner = x; console.log(
setter called: ${x}
); } What happens if i pass something in the "x" parameter?, what can i pass in it?. Breaking it down to simple terms helps me.Thank you for the help.